8 REST API Interview Questions Every Developer Should Know
REST APIs are fundamental in modern software development, playing a crucial role in building scalable, maintainable applications. As technology advances, the need for systems to communicate seamlessly with each other becomes paramount, and REST APIs stand at the forefront of this requirement. They allow different software to 'talk' to each other, transferring data in a format both can understand.
For developers, understanding REST APIs is essential due to their use cases in web services, cloud computing, and microservices architecture. A strong grasp of REST API principles enables developers to create more adaptable and efficient systems, which is a highly valued skill in today's tech landscape.
In this blog, we'll explore REST API fundamentals and their application in software development. Starting with a deep dive into REST API architecture, we'll then examine typical interview topics, from basic principles to complex scenarios. We will also cover practical interview questions complemented by pseudocode, providing insights into real-world implementation and enhancing your understanding for both interviews and practical development tasks.
What is a REST API?
An API, or Application Programming Interface, is a set of rules and protocols for building and interacting with software applications. REST, standing for Representational State Transfer, is a popular style of API used in web services.
Here are the key components of REST API:
- Resources: Accessed through unique URIs, they represent the specific data or functionality of the API.
- HTTP Methods: Methods like GET (retrieve data), POST (create new data), PUT (update existing data), and DELETE (remove data) define interactions with the resources.
- Stateless Interactions: Each request from a client contains all the information needed for the server to understand and respond, ensuring no client context is stored server-side.
REST APIs are ideal for creating scalable applications due to their stateless nature, which allows servers to process requests independently. This leads to benefits such as:
- Improved scalability by handling numerous simultaneous requests.
- Enhanced performance due to the stateless architecture and potential for caching.
- Simplicity in building and modifying the API, as each part can be developed independently.
What Could Be Asked in a REST API Interview?
In a REST API interview, the expectations from a candidate can vary but generally encompass a deep understanding of REST principles and the ability to apply them practically.
Here are challenges that the candidate could be asked to handle during the interview.
- Understanding of REST Principles: A thorough grasp of HTTP methods, URIs, statelessness, and other RESTful concepts.
- Practical Application: Demonstrating how these principles are applied in real-world scenarios.
- Problem-Solving Skills: Designing effective solutions for common REST API challenges.
- Debugging Tasks: Candidates might be given exercises to identify and resolve issues in existing API implementations.
- Coding Proficiency: Writing pseudocode or actual code, particularly focusing on designing and interacting with RESTful services.
- Performance Optimization: Understanding how to enhance the efficiency and scalability of APIs.
- Security Measures: Implement and maintain security protocols to protect data and services.
Top 8 REST API Interview Questions Every Developer Should Know
1. Create a Simple RESTful Service to Demonstrate CRUD Operations
This question will help you to understand CRUD operations and endpoint creation. It's a practical way to demonstrate your ability to build and manage a simple yet fundamental feature of web applications.
Question: Create a Flask API for managing a simple to-do list, implementing all CRUD (Create, Read, Update, Delete) operations.
Input Format: The API should accept JSON-formatted inputs for each CRUD operation, including details like to-do item descriptions and identifiers for updates and deletions.
Output Format: The API should return appropriate JSON-formatted responses indicating the success, failure, or results of the CRUD operations.
Code:
from flask import Flask, jsonify, request app = Flask(__name__) todos = [] # In-memory list to store to-do items # Route for handling both getting and adding to-do items @app.route('/todos', methods=['GET', 'POST']) def manage_todos(): if request.method == 'POST': # Adding a new to-do item todo = request.json todos.append(todo) return jsonify(todo), 201 # Returning all to-do items return jsonify(todos) # Route for updating and deleting a specific to-do item @app.route('/todos/<int:todo_id>', methods=['PUT', 'DELETE']) def update_delete_todo(todo_id): if request.method == 'PUT': # Updating an existing to-do item for todo in todos: if todo['id'] == todo_id: todo.update(request.json) return jsonify(todo) return jsonify({'error': 'Not found'}), 404 if request.method == 'DELETE': # Deleting a to-do item for i, todo in enumerate(todos): if todo['id'] == todo_id: del todos[i] return jsonify({'result': 'success'}) return jsonify({'error': 'Not found'}), 404 if __name__ == '__main__': app.run(debug=True)
In this code, the Flask framework is used to create a simple RESTful API. The manage_todos
function handles GET requests to retrieve all to-dos and POST requests to add a new to-do. The update_delete_todo
function handles PUT requests to update and DELETE requests to remove a specific to-do item, identified by its ID. The in-memory todos list acts as a simple database.
2. Implementing Basic Authentication
Implementing Basic Authentication is important to ensure that only authorized users can access certain API endpoints.
Question: Add basic authentication to a Flask API.
Input Format: The API request should contain a username and password to authenticate the user.
Output Format: The API should only allow access to authorized users and provide appropriate responses for both authenticated and unauthenticated requests.
Code:
from flask import Flask, request, jsonify from functools import wraps app = Flask(__name__) # Dummy user for demonstration users = {"user1": "password1"} # Basic authentication decorator def require_auth(f): @wraps(f) def decorated(*args, **kwargs): auth = request.authorization if not auth or users.get(auth.username) != auth.password: return jsonify({'message': 'Authentication required'}), 401 return f(*args, **kwargs) return decorated # Secure route requiring authentication @app.route('/secure-data') @require_auth def secure_data(): return jsonify({'data': 'Secure data accessed'}) if __name__ == '__main__': app.run(debug=True)
This code adds a basic authentication layer to the Flask app. The require_auth decorator
function checks for valid credentials in each request. If the request lacks valid authentication, it returns a 401 Unauthorized response. This demonstrates how to secure specific routes in a Flask application.
3. Implementing API Versioning
API versioning becomes essential when you need to introduce significant changes or improvements to an API without disrupting the service for existing clients. It's particularly important when modifications to the API would break compatibility with applications that rely on the older version. By implementing versioning, developers can ensure that both new and existing clients can use the API effectively, allowing for a smoother transition and better management of API updates over time.
Question: Implement versioning in a Flask API.
Input Format: The API should be able to distinguish and respond to requests based on the API version specified by the client.
Output Format: The API should return data according to the version of the API that is being requested.
Code:
from flask import Flask, jsonify app = Flask(__name__) # Version 1 of the API @app.route('/v1/data') def data_v1(): return jsonify({'data': 'Version 1 data'}) # Version 2 of the API, with changes @app.route('/v2/data') def data_v2(): return jsonify({'data': 'Version 2 data with additional feature'}) if __name__ == '__main__': app.run(debug=True)
In this code, two different endpoints are created, each representing a different version of the API (v1 and v2). This approach allows clients to specify the version they wish to interact with, ensuring backward compatibility and easier transition to newer API versions.
4. Custom HTTP Status Codes and Error Handling
Proper error handling in APIs, including the use of custom HTTP status codes, is crucial for clear communication between the server and the client. It allows the server to inform clients not only about the success or failure of a request but also about the problem with a clear message. This is especially important for diagnosing issues, improving user experience, and ensuring the API is robust and reliable.
Question: Implement custom HTTP status codes and error handling in a Flask API.
Input Format: The API should identify different types of errors or exceptions and respond accordingly.
Output Format: The API should return custom error messages and appropriate HTTP status codes based on the error type.
Code:
from flask import Flask, jsonify, make_response app = Flask(__name__) @app.route('/some-endpoint') def some_endpoint(): try: # API logic here pass except SomeSpecificError as e: return make_response(jsonify({'error': str(e)}), 400) except AnotherError as e: return make_response(jsonify({'error': str(e)}), 404) # More exceptions can be handled here return make_response(jsonify({'result': 'success'}), 200) @app.errorhandler(404) def not_found(error): return make_response(jsonify({'error': 'Not found'}), 404) if __name__ == '__main__': app.run(debug=True)
This code includes an example of handling different types of errors within an API endpoint and sending appropriate HTTP responses. The errorhandler
decorator is used for handling global errors like 404 Not Found. This setup ensures that the client receives both a clear error message and a relevant HTTP status code, aiding in debugging and user experience.
5. Implementing Rate Limiting
Implementing rate limiting in an API is crucial for controlling traffic and preventing overuse or abuse of the API. It's a key aspect of API management, ensuring the service remains reliable and performs optimally even under high load.
Question: Add rate limiting to a Flask API.
Input Format: The API should monitor the number of requests from a user or IP address over a defined period.
Output Format: The API should limit access when a user exceeds the predefined request limit, returning a message indicating the rate limit has been exceeded.
Code:
from flask import Flask, request, jsonify, make_response from flask_limiter import Limiter from flask_limiter.util import get_remote_address # Initialize Flask app app = Flask(__name__) # Initialize Limiter for rate limiting limiter = Limiter(app, key_func=get_remote_address) # Define a route with rate limiting @app.route('/limited-access') @limiter.limit("5 per minute") # Limit to 5 requests per minute def limited_access(): # Respond to valid requests return jsonify({'response': 'This route is rate-limited'}) # Custom error handler for rate limit exceeded @app.errorhandler(429) def ratelimit_handler(e): # Respond with error message when rate limit is exceeded return make_response(jsonify({'error': 'Rate limit exceeded'}), 429) # Run the Flask app if __name__ == '__main__': app.run(debug=True)
This code sets up basic rate limiting using flask_limiter. The @limiter.limit
decorator specifies the rate limit for the endpoint. If the limit is exceeded, a 429 Too Many Requests error is returned, handled by the ratelimit_handler
function. This ensures the API usage is controlled and prevents abuse.
6. Design an API Endpoint for User Registration
Designing an API endpoint for user registration and password updates involves handling sensitive user information securely. This question tests your ability to create secure and efficient user management within an API.
Question: Create a Flask API endpoint for user registration and password updates.
Input Format: The API should accept user details like email and password for registration, and for password updates, it should require the user's email and new password.
Output Format: The API should return a confirmation message for successful registration or password update, along with appropriate status codes.
Code:
from flask import Flask, request, jsonify app = Flask(__name__) users = {} # Dictionary to store user data # Route to handle user registration @app.route('/register', methods=['POST']) def register(): # Get JSON data sent with POST request user_data = request.json email = user_data.get('email') # Check if the user already exists if email in users: # User exists, return conflict error return jsonify({'message': 'User already exists'}), 409 # Add new user to the dictionary users[email] = user_data.get('password') # Return success message return jsonify({'message': 'User registered successfully'}), 201 # Route to update user's password @app.route('/update-password', methods=['PUT']) def update_password(): # Get JSON data sent with PUT request user_data = request.json email = user_data.get('email') # Check if the user exists if email not in users: # User not found, return not found error return jsonify({'message': 'User not found'}), 404 # Update user's password users[email] = user_data.get('new_password') # Return success message return jsonify({'message': 'Password updated successfully'}), 200 # Run the Flask app if __name__ == '__main__': app.run(debug=True)
This code provides a simple implementation of user registration and password updates. The register
route adds a new user to the users dictionary, while the update-password
route updates an existing user's password. The example prioritizes simplicity and clarity to demonstrate the basic mechanics of handling user data in a Flask API.
7. Implementing Pagination in a REST API
Implementing pagination using a REST API is essential for handling large datasets efficiently. This task tests your ability to manage and present data in a user-friendly and resource-efficient manner.
Question: Add pagination functionality to a Flask API.
Input Format: The API should accept parameters to control the page number and the number of items per page.
Output Format: The API should return a portion of the dataset corresponding to the specified page, along with information about the total number of pages.
Code:
from flask import Flask, request, jsonify app = Flask(__name__) data = [...] # A large list of data, replace with actual data @app.route('/data') def get_paginated_data(): # Retrieve page number and number of items per page from query parameters page = int(request.args.get('page', 1)) # Default to first-page per_page = int(request.args.get('per_page', 10)) # Default to 10 items per page # Calculate start and end indices for the slice start = (page - 1) * per_page end = start + per_page # Slice the data to get the requested page paginated_data = data[start:end] # Calculate the total number of pages total_pages = len(data) // per_page + (1 if len(data) % per_page else 0) # Return the paginated data and the total number of pages return jsonify({'data': paginated_data, 'total_pages': total_pages}) if __name__ == '__main__': app.run(debug=True)
This code demonstrates how to implement basic pagination in a Flask API. The get_paginated_data
function takes query parameters for the page number and the number of items per page, slicing the data accordingly. It also calculates and returns the total number of pages. This approach is essential for efficient data retrieval and display in applications handling large datasets.
8. Implement Filtering and Sorting in a RESTful API
Implementing filtering and sorting in a RESTful API is essential for enhancing data retrieval efficiency and user experience, especially in data-rich applications.
Question: Add filtering and sorting capabilities to a Flask API.
Input Format: The API should accept query parameters for filtering and sorting criteria (e.g., filter by category, sort by date).
Output Format: The API should return data that matches the filtering criteria and is sorted according to the specified parameters.
Code
from flask import Flask, request, jsonify app = Flask(__name__) data = [...] # Replace with your dataset @app.route('/items') def get_items(): # Filtering filter_category = request.args.get('category') filtered_data = [item for item in data if item['category'] == filter_category] if filter_category else data # Sorting sort_by = request.args.get('sort_by', 'date') # Default sorting by date reverse_sort = True if request.args.get('order') == 'desc' else False sorted_data = sorted(filtered_data, key=lambda x: x[sort_by], reverse=reverse_sort) return jsonify(sorted_data) if __name__ == '__main__': app.run(debug=True)
In this code, the API endpoint /items
allows for filtering and sorting of data. Query parameters determine the category to filter by and the attribute to sort by, with an option for ascending or descending order. This implementation showcases how to dynamically handle user requests for specific data views in a Flask API.
Final Thoughts
REST APIs are integral to modern software development, enabling seamless communication between systems. This blog has provided a comprehensive overview of REST API concepts, from basic principles to advanced implementations using Flask. By understanding these concepts and mastering the practical examples, developers can enhance their skills in creating efficient, scalable, and secure web services. Whether preparing for interviews or developing real-world applications, these insights into REST APIs are invaluable for any aspiring or experienced software developer.
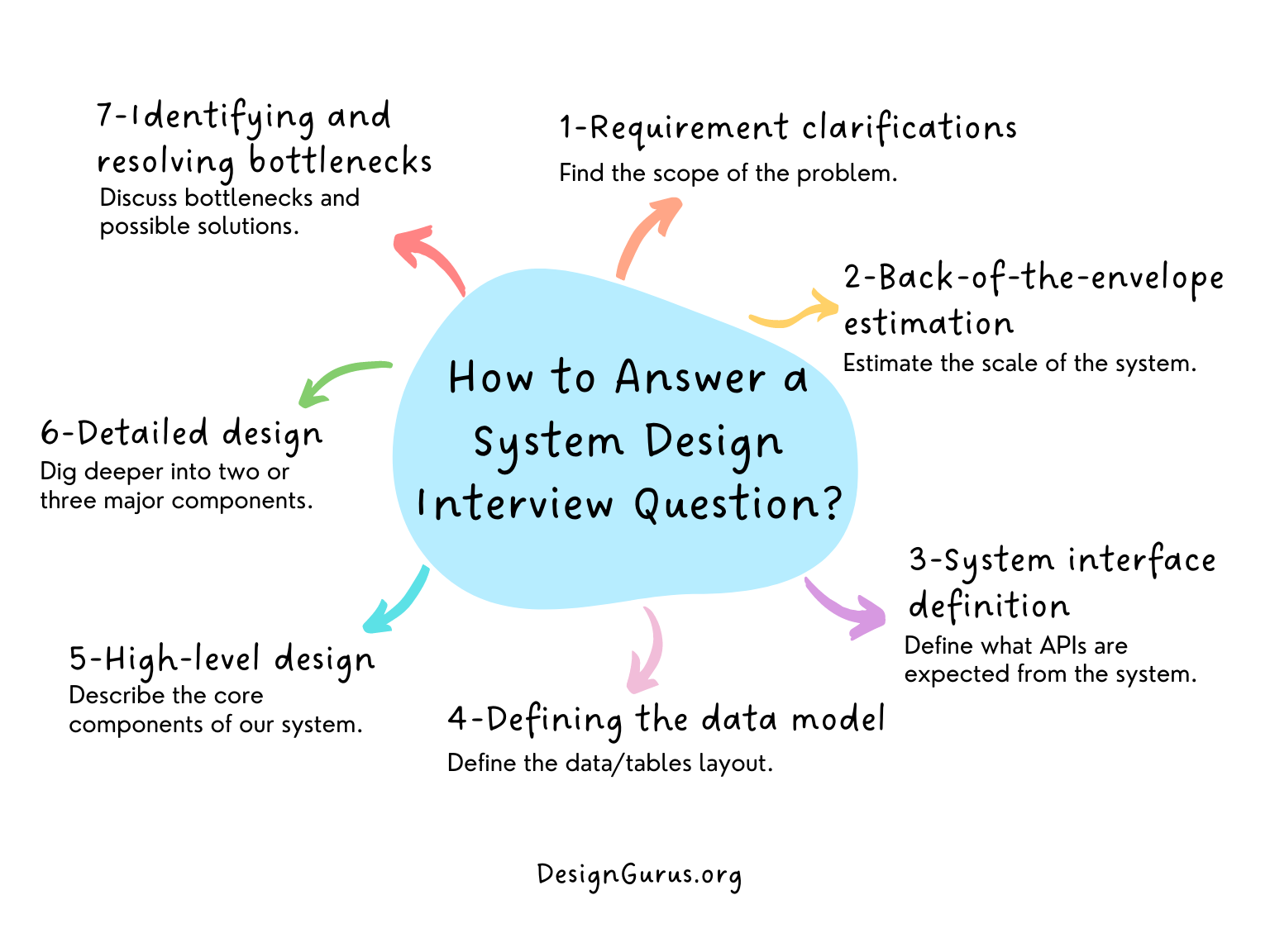
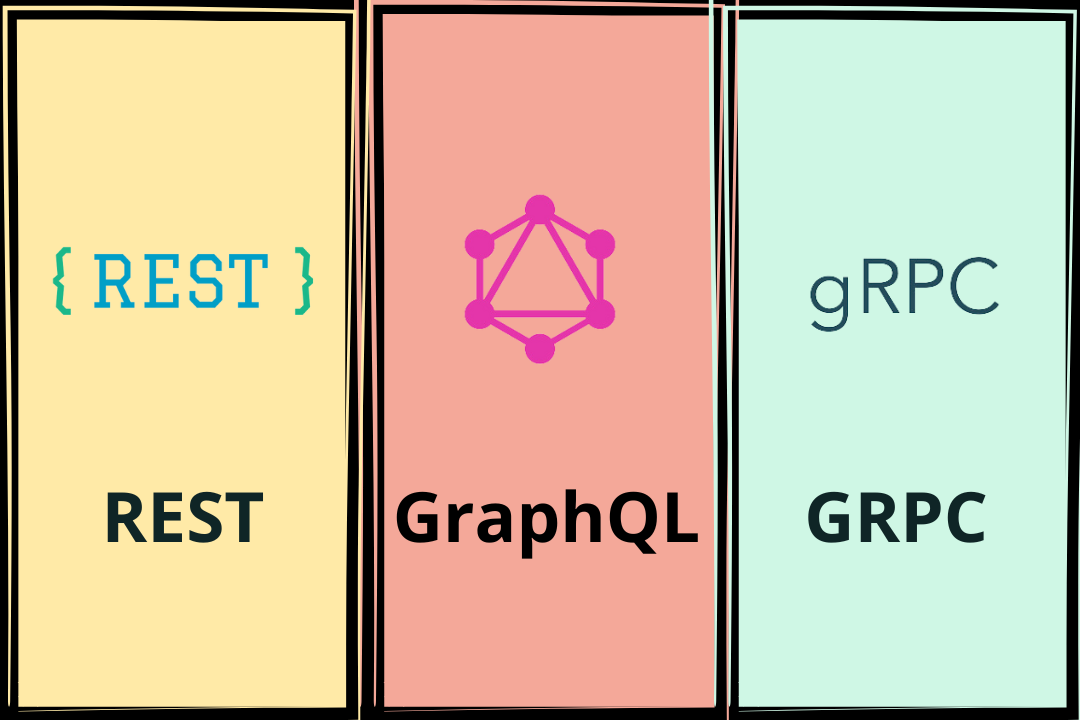