Essential Software Design Principles You Should Know Before the Interview
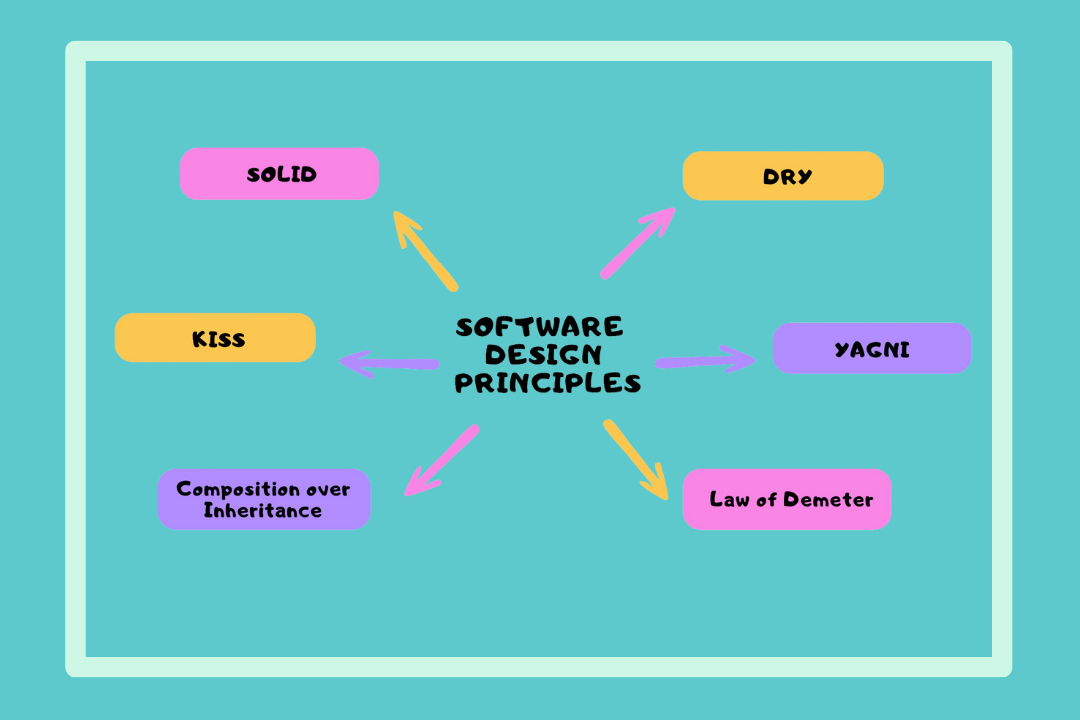
Software design principles are a collection of guidelines, best practices, and concepts that assist developers in creating well-structured, maintainable, and efficient software systems. These principles provide a solid foundation for writing code that is easy to understand, modify, and extend, ultimately leading to improved software quality.
The key objectives of software design principles include:
- Encouraging code reusability and modularity.
- Promoting loose coupling between components or modules.
- Ensuring code readability and understandability.
- Enhancing testability, maintainability, and scalability.
- Facilitating effective collaboration among team members.
Several well-known software design principles are:
- SOLID: A set of five object-oriented design principles that focus on creating maintainable and scalable code.
- DRY (Don't Repeat Yourself): A principle that emphasizes avoiding code duplication to reduce maintenance complexity and potential inconsistencies.
- KISS (Keep It Simple, Stupid): A principle that encourages simplicity in code and design, avoiding unnecessary complexity.
- YAGNI (You Aren't Gonna Need It): A principle that advises developers to focus on delivering the features that are currently needed, rather than over-engineering or anticipating future requirements.
- Composition Over Inheritance: A design principle that encourages the use of composition (combining simple objects to create more complex ones) instead of inheritance (creating new classes by inheriting properties and methods from a parent class) for code reuse and extensibility.
- Law of Demeter (Principle of Least Knowledge): A principle that promotes reducing coupling between components by limiting the knowledge an object has about other objects in the system.
SOLID Principles
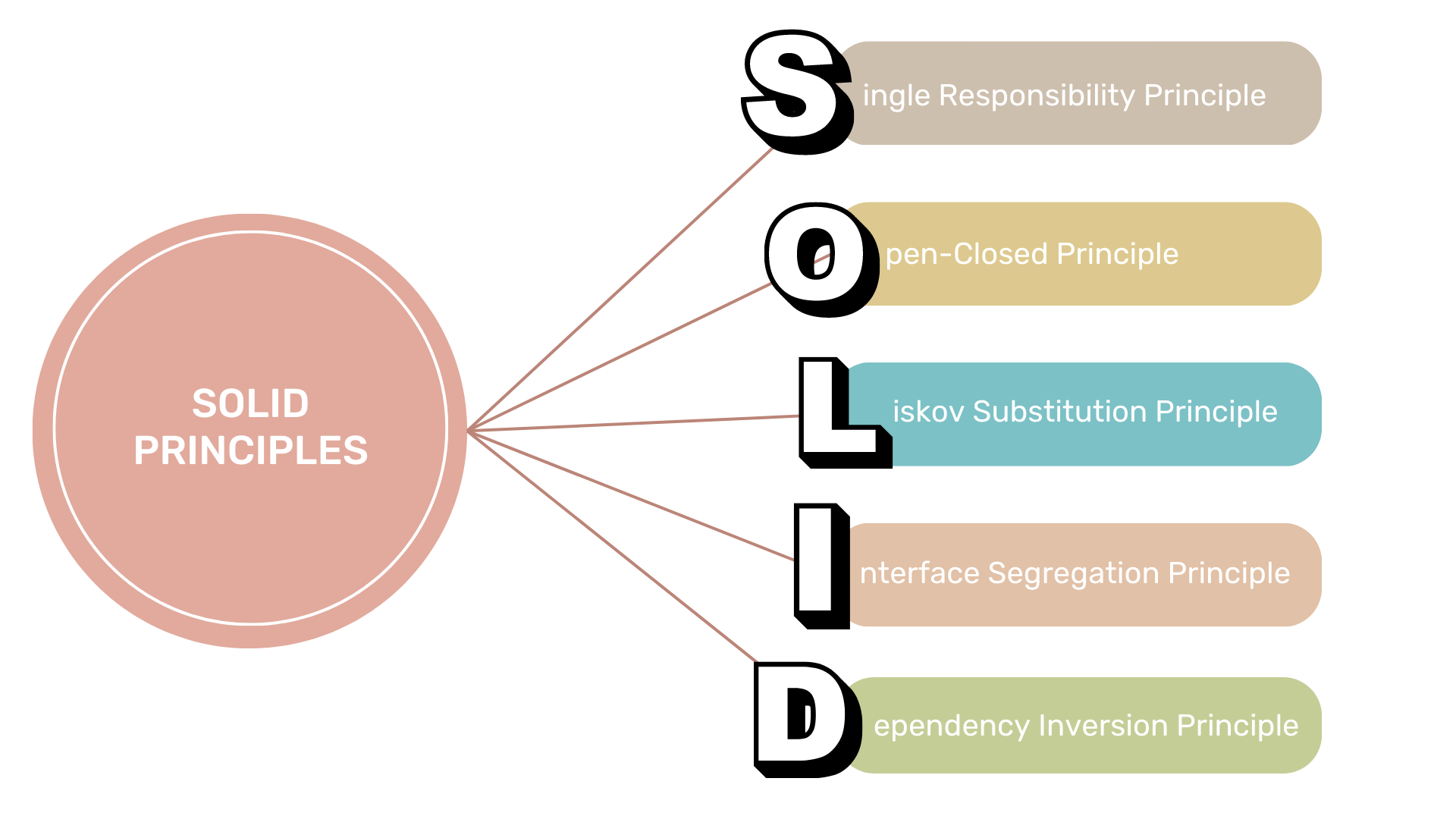
SOLID is a set of design principles that guide developers in creating maintainable, scalable, and efficient object-oriented software systems. These principles provide a foundation for writing code that is easy to understand, modify, and extend. By adhering to SOLID principles, developers can create a well-structured codebase that is less prone to bugs and easier to refactor. SOLID principles not only improve the overall quality of the software but also make it more adaptable to changing requirements and facilitate collaboration among team members.
SOLID is an acronym that represents five fundamental principles:
- Single Responsibility Principle (SRP)
- Open-Closed Principle (OCP)
- Liskov Substitution Principle (LSP)
- Interface Segregation Principle (ISP)
- Dependency Inversion Principle (DIP)
Now let's briefly explain other software design principles:
Single Responsibility Principle (SRP)
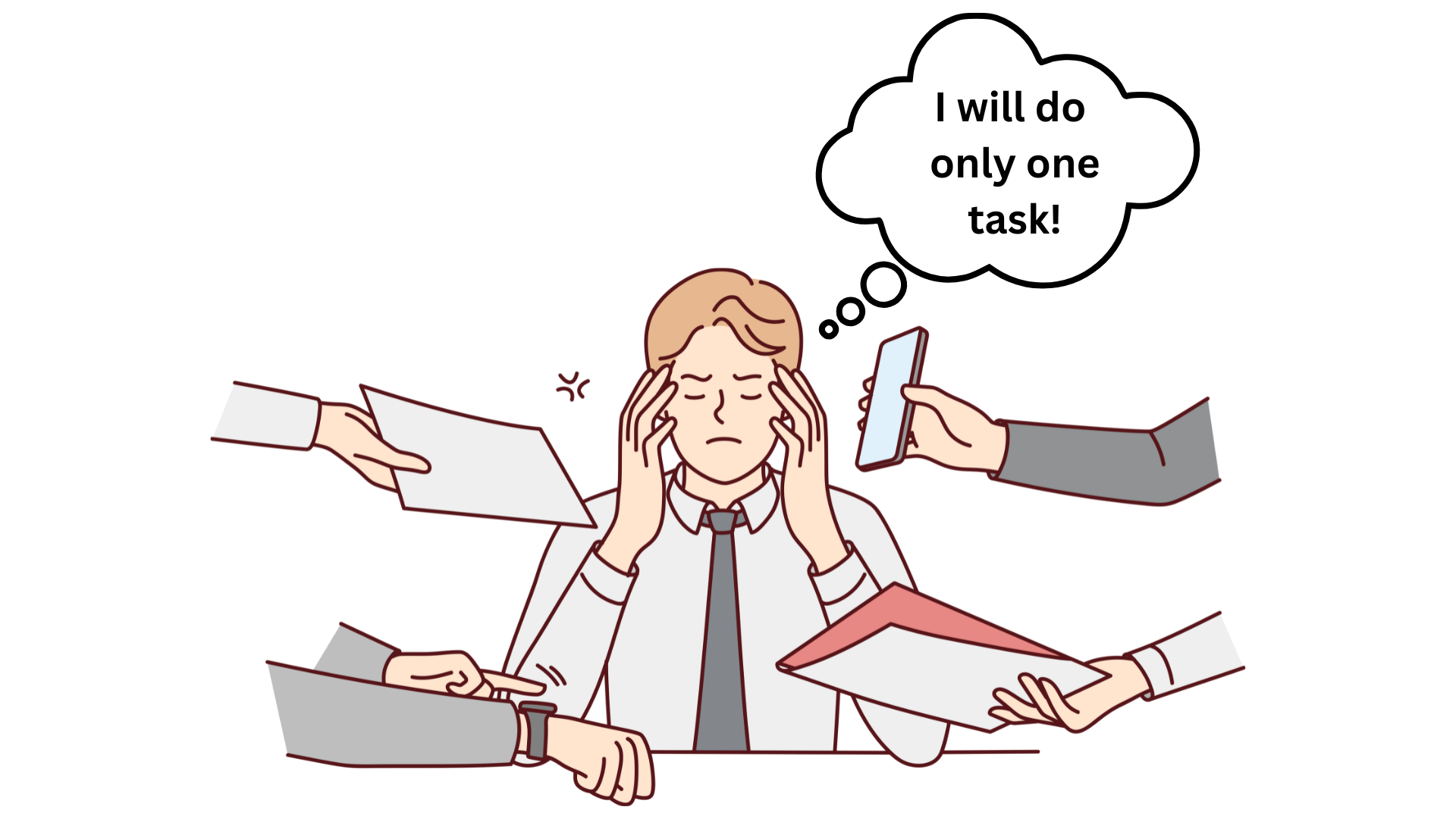
Definition: The Single Responsibility Principle states that a class should have only one reason to change, meaning it should have just one responsibility. In other words, each class should focus on a single task or concern to make the code more maintainable and understandable.
Benefits:
- Easier to maintain and understand the code: By focusing on a single responsibility, each class becomes simpler, making it easier to comprehend and modify.
- Reduced coupling between classes: Since each class has a single responsibility, it's less likely to be tightly coupled to other classes. This makes the overall codebase more flexible.
- Increased cohesion within a class: A class with a single responsibility will have a higher degree of cohesion since all its methods and properties are closely related to its primary task.
Example:
Let's consider a scenario where you're building a content management system (CMS) that involves user management, content creation, and content publishing. Here's how you can apply the SRP to create classes with a single responsibility:
// Not the best design class CMS { createUser() { // Create a new user } deleteUser() { // Delete a user } createContent() { // Create new content } editContent() { // Edit existing content } publishContent() { // Publish content } } // A much better design, adhering to the SRP class UserManager { createUser() { // Create a new user } deleteUser() { // Delete a user } } class ContentManager { createContent() { // Create new content } editContent() { // Edit existing content } } class ContentPublisher { publishContent() { // Publish content } }
By following the Single Responsibility Principle, you can design classes that are easier to understand, maintain, and modify. Remember that while this principle is a useful guideline, you should always consider the specific context of your project and strike a balance between simplicity and over-fragmentation of responsibilities.
Open-Closed Principle (OCP)
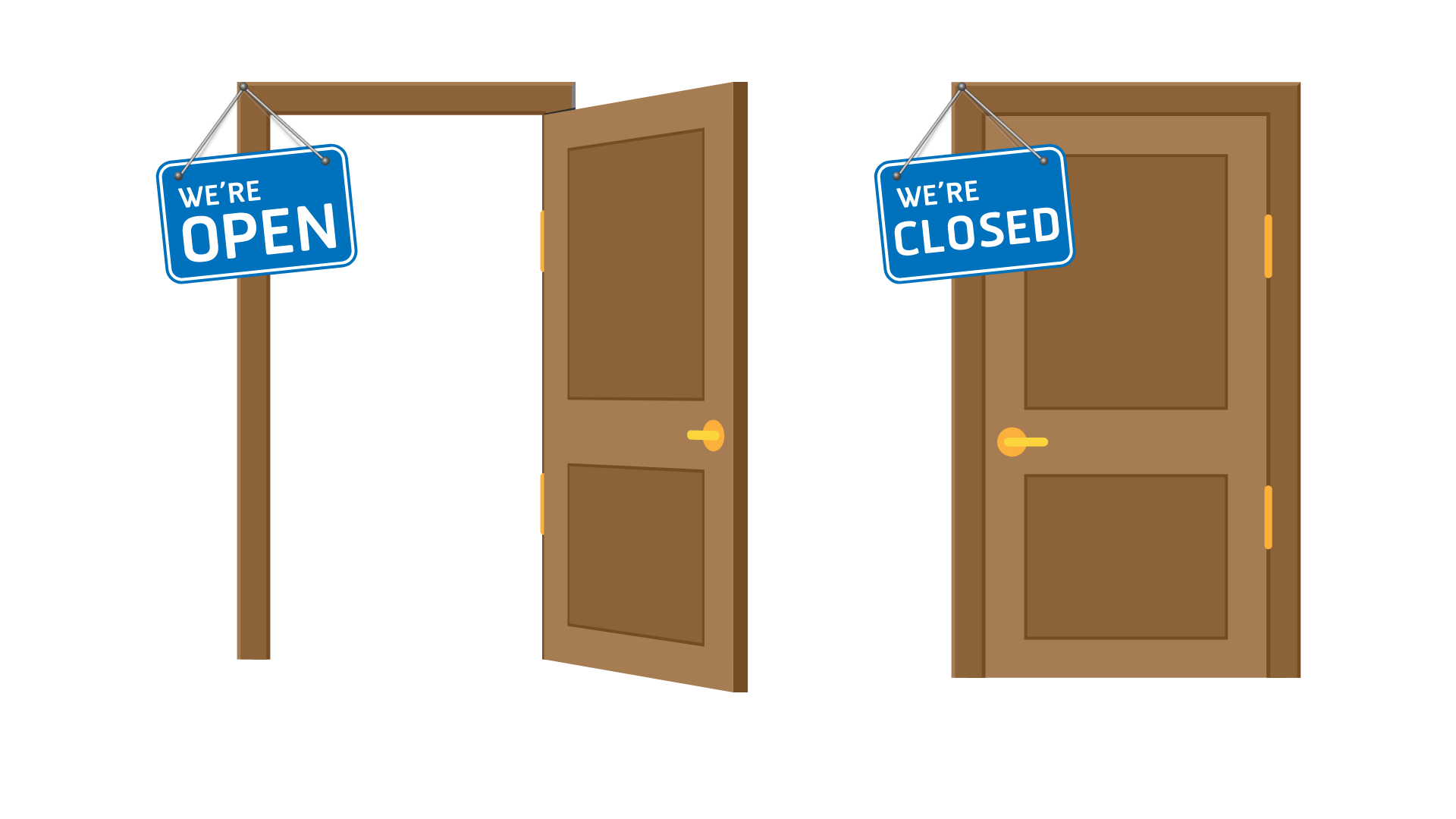
Definition: The Open-Closed Principle states that software entities (classes, modules, functions, etc.) should be open for extension but closed for modification. In other words, you should be able to add new functionality to a class without modifying its existing code, by extending it or using other mechanisms like composition.
Benefits:
- Increased flexibility: Adhering to OCP makes it easier to add new features without the risk of breaking existing functionality.
- Improved maintainability: Since you don't need to modify existing code to add new features, there's a lower chance of introducing bugs or regression issues.
- Enhanced modularity: Following OCP encourages modular design, making your codebase more organized and easier to manage.
Example:
Suppose you're building a system that calculates the area of different shapes like circles and rectangles. Here's how you can apply the Open-Closed Principle to create a flexible and maintainable design:
// Not the best design class AreaCalculator { calculateArea(shape) { if (shape instanceof Circle) { return Math.PI * shape.radius * shape.radius; } else if (shape instanceof Rectangle) { return shape.width * shape.height; } // Adding a new shape would require modifying this method } } // A much better design, adhering to the OCP class Shape { calculateArea() { // Default implementation, can be overridden in derived classes } } class Circle extends Shape { constructor(radius) { super(); this.radius = radius; } calculateArea() { return Math.PI * this.radius * this.radius; } } class Rectangle extends Shape { constructor(width, height) { super(); this.width = width; this.height = height; } calculateArea() { return this.width * this.height; } } class AreaCalculator { calculateArea(shape) { return shape.calculateArea(); } }
By following the Open-Closed Principle, you can design classes that are flexible and easy to maintain. Keep in mind that it's a guideline, not a strict rule. Sometimes, it's necessary to modify existing code to improve it or fix issues. The key is to strike a balance between keeping your code flexible and maintaining its quality.
Liskov Substitution Principle (LSP)
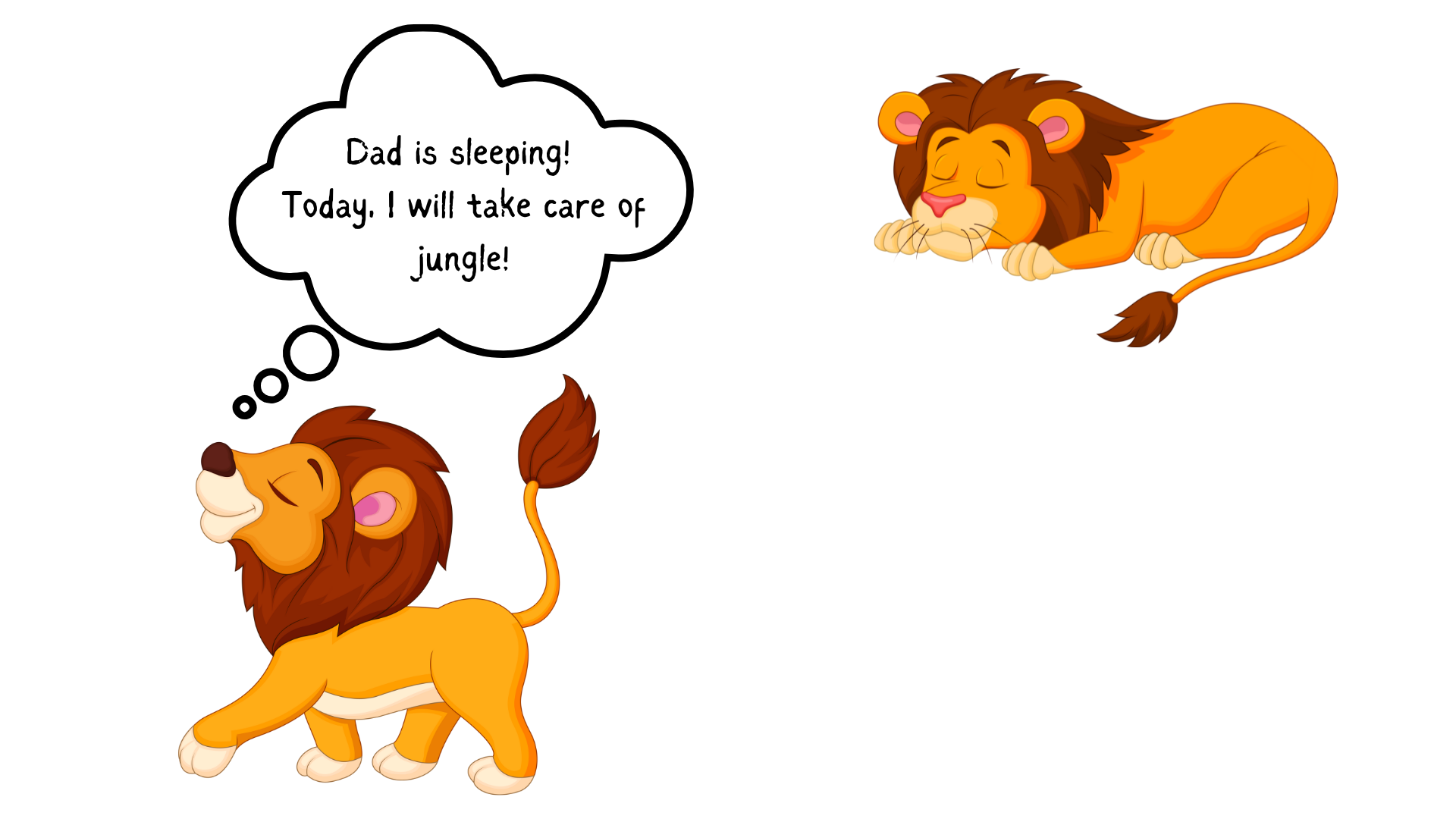
Definition: The Liskov Substitution Principle states that objects of a derived class should be able to replace objects of the base class without affecting the program's correctness. In other words, derived classes should adhere to the behavior and contracts defined by the base class.
Benefits:
- Improved code reusability: By ensuring that derived classes can be substituted for their base classes, you create a more flexible and reusable codebase.
- Enhanced maintainability: LSP helps you maintain the consistency of class hierarchies, making it easier to reason about the behavior of derived classes.
- Better abstractions: By adhering to LSP, you create more robust abstractions, reducing the likelihood of introducing bugs or unintended behavior.
Example:
Imagine you're building a system to manage a zoo, with classes to represent different types of birds. Here's how you can apply the Liskov Substitution Principle to design a class hierarchy that respects the expected behavior of birds:
// Base class class Bird { fly() { // Default implementation for flying } layEggs() { // Default implementation for laying eggs } } // Derived class class Eagle extends Bird { fly() { // Eagle-specific flying behavior } layEggs() { // Eagle-specific egg-laying behavior } } // Incorrect derived class - violates LSP class Penguin extends Bird { // Penguins cannot fly, so the fly method shouldn't be inherited or implemented } // A better design adhering to LSP class FlightlessBird extends Bird { // Remove or override the fly method as it's not applicable to flightless birds fly() { throw new Error("This bird cannot fly."); } } class Penguin extends FlightlessBird { // Penguin-specific egg-laying behavior layEggs() { // ... } }
By following the Liskov Substitution Principle, you can design class hierarchies that are consistent, maintainable, and reusable. Remember that LSP is a guideline to help you create better abstractions and more reliable code, but it's essential to consider the specific context of your project to apply it effectively.
Interface Segregation Principle (ISP)
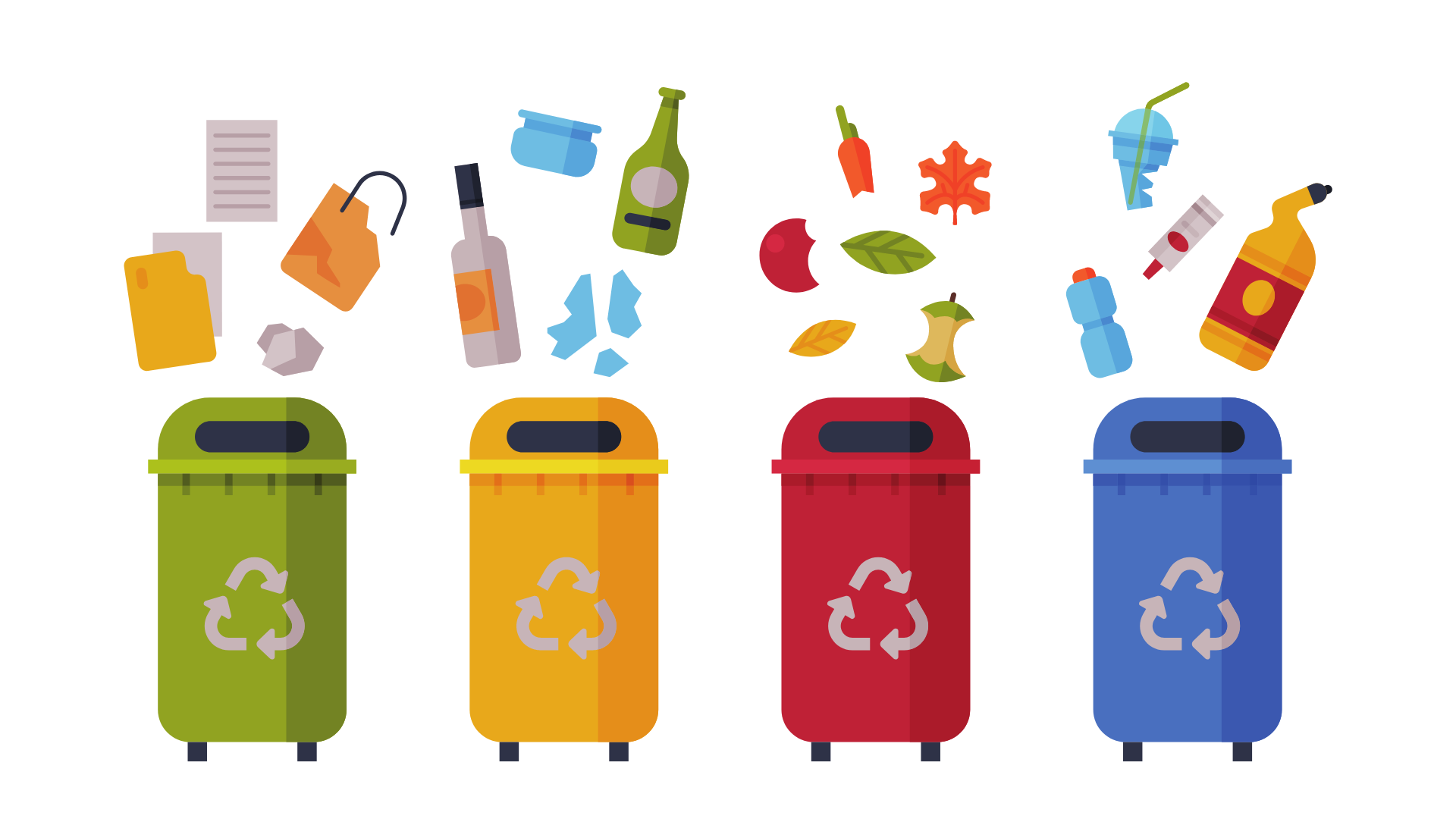
Definition: The Interface Segregation Principle states that clients should not be forced to depend on interfaces they do not use. In other words, large interfaces should be split into smaller, more specific ones so that a class implementing the interface only needs to focus on methods that are relevant to its functionality.
Benefits:
- Reduced coupling between classes: By splitting interfaces into smaller, more focused ones, you minimize the dependencies between classes, leading to a more flexible and maintainable codebase.
- Increased code readability: Smaller interfaces are easier to understand and implement, making your code more readable.
- Easier to update and modify interfaces: Since the interfaces are more focused, they're less likely to change, making it easier to implement and update them without breaking existing code.
Example:
Suppose you're building a system that involves various types of devices with different capabilities, such as printers, scanners, and fax machines. Here's how you can apply the Interface Segregation Principle to create focused interfaces for each device:
// Not the best design - too many unrelated methods in a single interface class AllInOneDevice { print() { // Print implementation } scan() { // Scan implementation } fax() { // Fax implementation } } // A much better design, adhering to the ISP class Printer { print() { // Print implementation } } class Scanner { scan() { // Scan implementation } } class FaxMachine { fax() { // Fax implementation } } // Now, a class can implement only the interfaces it needs class SimplePrinter extends Printer { print() { // Simple printing implementation } } class AllInOnePrinter extends Printer, Scanner, FaxMachine { print() { // Advanced printing implementation } scan() { // Advanced scanning implementation } fax() { // Advanced faxing implementation } }
By following the Interface Segregation Principle, you can design classes and interfaces that are more focused, maintainable, and flexible. It's essential to consider the specific context of your project and strike a balance between creating focused interfaces and not over-fragmenting your interfaces.
Dependency Inversion Principle (DIP)
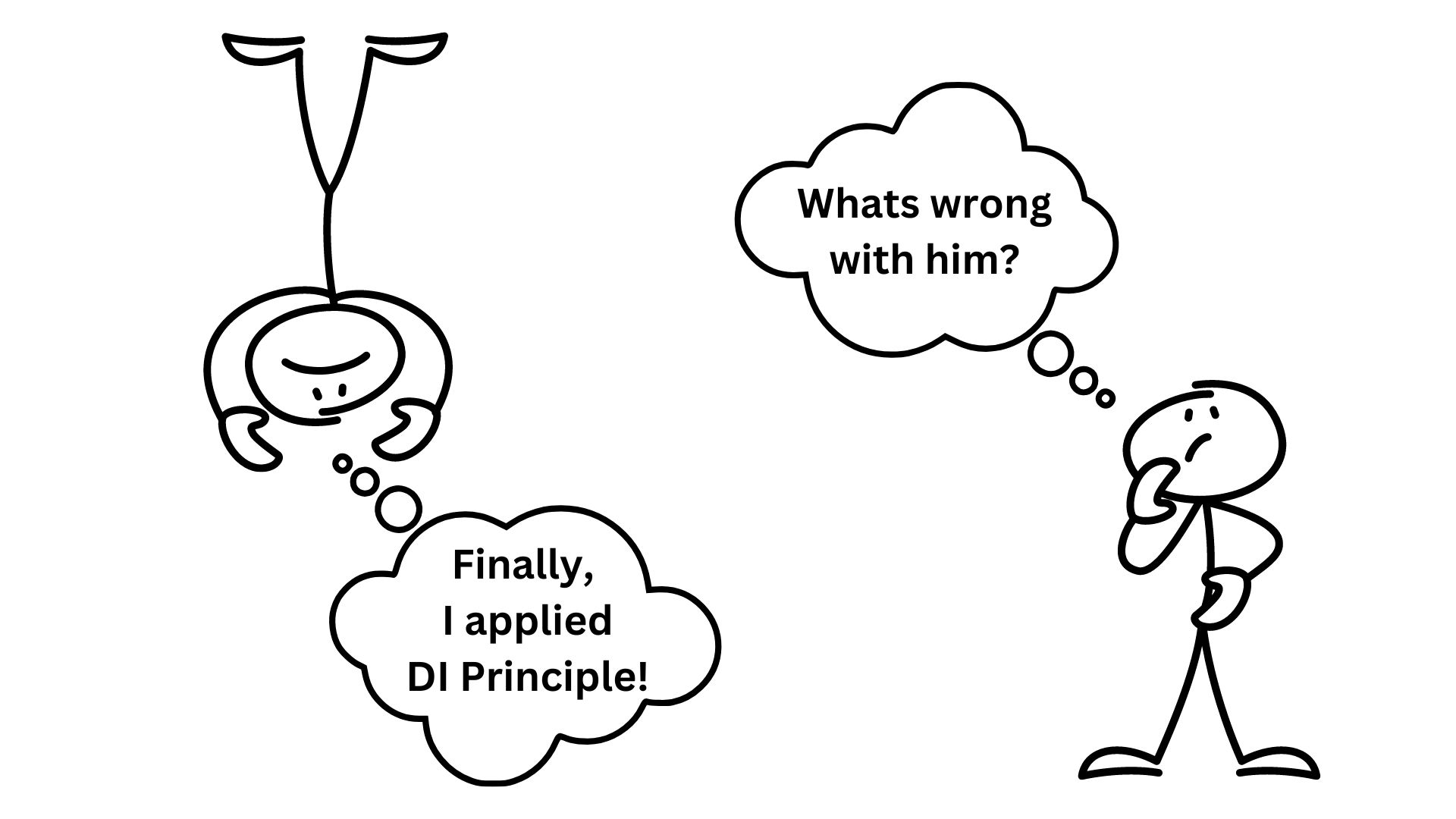
Definition: The Dependency Inversion Principle states that high-level modules should not depend on low-level modules; both should depend on abstractions. Furthermore, abstractions should not depend on details; details should depend on abstractions. In simple terms, this principle encourages you to depend on abstract interfaces rather than concrete implementations, promoting loose coupling and better separation of concerns.
Benefits:
- Increased flexibility: By depending on abstractions, you can easily change or swap concrete implementations without affecting high-level modules, making your code more flexible.
- Improved maintainability: Loosely coupled code is easier to maintain and modify since changes in one module are less likely to impact other modules.
- Enhanced testability: By depending on abstractions, you can mock or stub dependencies during testing, making it easier to write and maintain tests.
Example:
Consider a scenario where you're building an application that sends notifications to users through different channels like email or SMS. Here's how you can apply the Dependency Inversion Principle to create a flexible and maintainable design:
// Not the best design class EmailNotifier { sendEmail(message) { // Send email implementation } } class SMSNotifier { sendSMS(message) { // Send SMS implementation } } class NotificationService { constructor() { this.emailNotifier = new EmailNotifier(); this.smsNotifier = new SMSNotifier(); } sendNotification(message) { this.emailNotifier.sendEmail(message); this.smsNotifier.sendSMS(message); // Adding a new notifier would require modifying this class } } // A much better design, adhering to the DIP class Notifier { send(message) { // Default implementation, can be overridden in derived classes } } class EmailNotifier extends Notifier { send(message) { // Send email implementation } } class SMSNotifier extends Notifier { send(message) { // Send SMS implementation } } class NotificationService { constructor(notifiers) { this.notifiers = notifiers; } sendNotification(message) { this.notifiers.forEach(notifier => { notifier.send(message); }); } }
By following the Dependency Inversion Principle, you can create code that is more flexible, maintainable, and testable. Remember that this principle is a guideline to help you create better abstractions and reduce coupling, but you should always consider the specific context of your project to apply it effectively.
DRY (Don't Repeat Yourself)
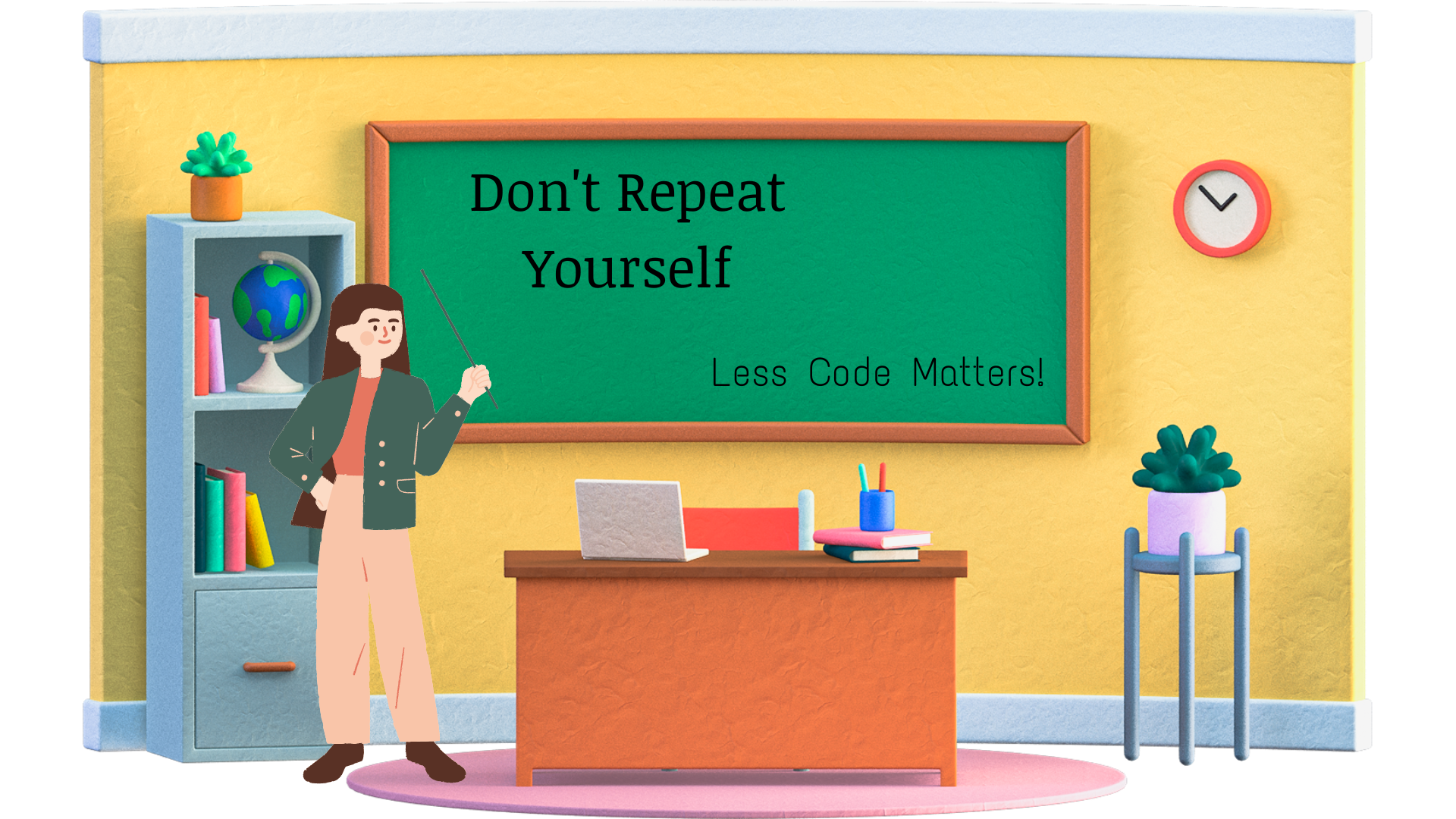
Definition: The DRY principle is a software development guideline that encourages developers to avoid duplicating code and logic. It emphasizes the importance of reusing code through abstractions, modularization, and encapsulation. By following the DRY principle, you can create a codebase that is easier to maintain, modify, and extend.
Benefits:
• Easier maintenance: When you need to make changes or fix bugs, you only have to do it in one place, reducing the risk of introducing inconsistencies or errors.
• Improved readability: By eliminating duplication, you can make the code more concise and easier to understand.
• Faster development: Reusing code allows you to build features more quickly and reduces the amount of code you need to write and maintain.
Techniques to achieve DRY code:
- Functions and methods: Encapsulate repeated logic within functions or methods that can be called from multiple places.
- Inheritance and composition: Reuse common functionality through inheritance or composition, avoiding the duplication of code across classes or components.
- Modularization: Break your code into smaller, reusable modules or libraries that can be imported and used in different parts of the application.
- Design patterns: Apply established design patterns that promote reusability and avoid duplication.
- Code refactoring: Continuously review and refactor your code to identify and eliminate repetitions.
Example:
Suppose you're building an application that calculates the area of different shapes. You might find yourself repeatedly using the formula to calculate the area of a rectangle. Here's how applying the DRY principle can help you avoid duplicating code:
// Not adhering to DRY - repeated logic to calculate the area of a rectangle function calculateAreaOfSquare(side) { return side * side; // Repeated logic } function calculateAreaOfRectangle(length, width) { return length * width; // Repeated logic } // Adhering to DRY - encapsulate the repeated logic in a function function calculateAreaOfRectangle(length, width) { return length * width; } function calculateAreaOfSquare(side) { return calculateAreaOfRectangle(side, side); }
By following the DRY principle, you can create code that is easier to maintain, read, and extend. Keep in mind that while it's important to avoid unnecessary duplication, it's also essential not to over-engineer your solution in pursuit of reusability. Striking a balance between DRY and readability is key to creating maintainable and efficient code.
KISS (Keep It Simple, Stupid)
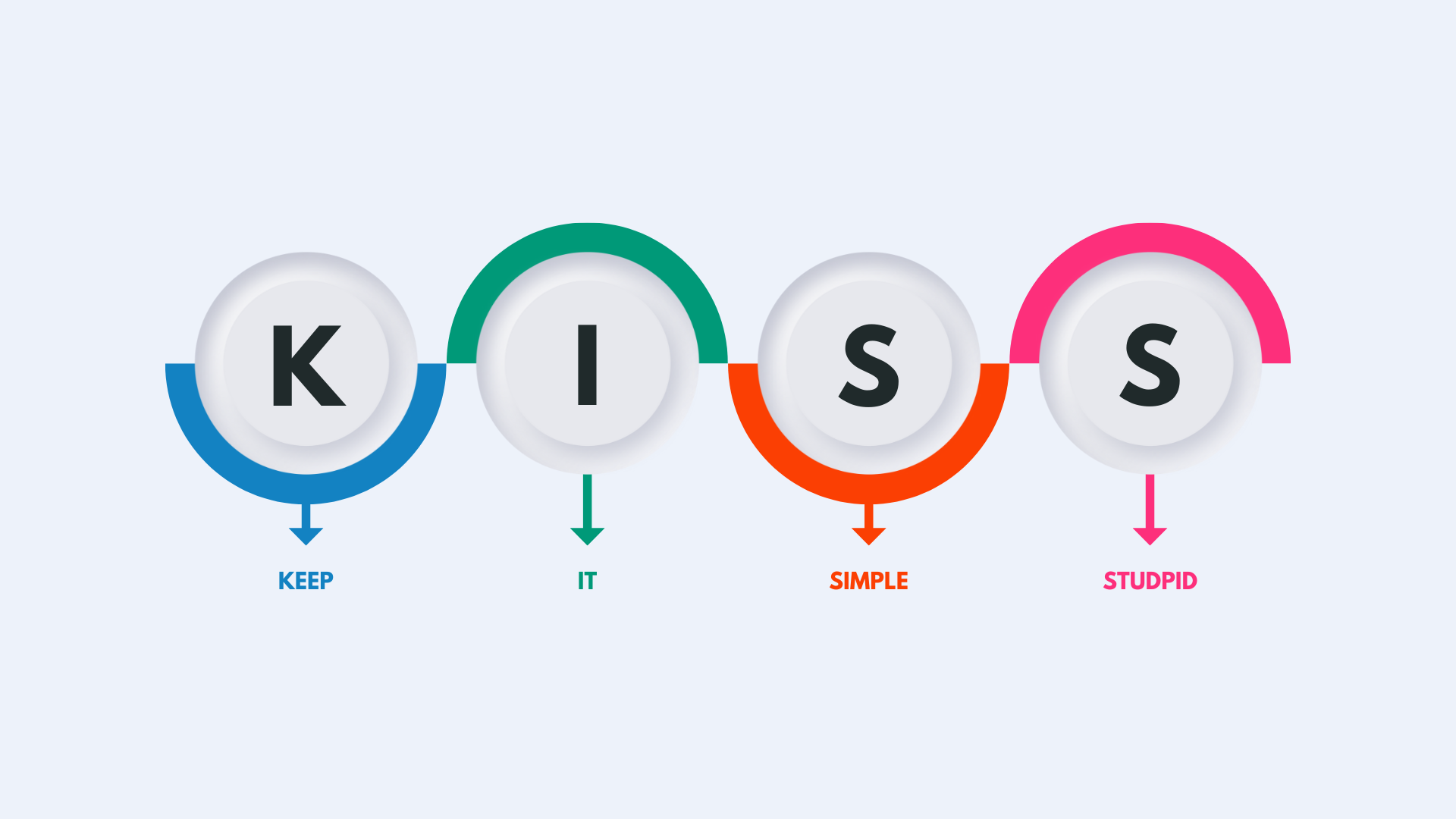
Definition: The KISS principle is a software development guideline that encourages developers to keep their solutions as simple as possible. It's based on the idea that the simplest solution is often the best, and unnecessary complexity should be avoided. The KISS principle aims to make code easier to understand, maintain, and modify.
Benefits:
- Improved code readability: Simple code is easier to read, understand, and debug, making it more maintainable in the long run.
- Faster development: By focusing on simplicity, you can often build features more quickly and with fewer bugs.
- Easier collaboration: Simple code is easier for other team members to work with, leading to better collaboration and knowledge sharing.
When to apply KISS:
The KISS principle should be applied throughout the software development process, from design to implementation. It's particularly useful when:
- Designing code architecture, ensuring it's easy to understand and modify.
- Writing code, by choosing straightforward algorithms and data structures.
- Refactoring code, by simplifying complex logic and removing unnecessary components.
Example:
Imagine you're building a function that calculates the sum of all the even numbers in an array. Here's how applying the KISS principle can help you write clean and simple code:
// Not adhering to KISS - overly complex solution function sumEvenNumbers(arr) { return arr .filter((num) => num % 2 === 0) .reduce((accumulator, currentValue) => accumulator + currentValue, 0); } // Adhering to KISS - a simpler and more straightforward solution function sumEvenNumbers(arr) { let sum = 0; for (let i = 0; i < arr.length; i++) { if (arr[i] % 2 === 0) { sum += arr[i]; } } return sum; }
By following the KISS principle, you can create code that is easier to understand, maintain, and collaborate on. Keep in mind that simplicity is a relative concept, and what's simple for one person may not be for another. The key is to strive for code that's easy to understand and work with, without compromising on functionality or performance.
YAGNI (You Aren't Gonna Need It)
Definition: YAGNI is a software development principle that encourages developers to focus on implementing only the features and functionalities that are required at the moment, rather than attempting to predict future requirements. The principle is based on the idea that it's better to add functionality as it's needed, rather than trying to anticipate and build for future needs that may never arise.
Benefits:
- Faster development: By focusing only on current requirements, you can deliver features more quickly and reduce development time.
- Reduced complexity: Implementing only what's needed helps keep the codebase simple, making it easier to understand and maintain.
- Minimized waste: By avoiding unnecessary features, you reduce wasted effort and resources on functionality that may never be used.
When to apply YAGNI:
YAGNI is particularly useful in Agile development methodologies, where the focus is on iterative and incremental development. It should be applied when:
- The future requirements are uncertain or subject to change.
- You are working on a tight schedule and need to prioritize the most important features.
- You want to minimize the risk of overengineering and code bloat.
Example:
Suppose you're building an e-commerce platform that supports creating and managing orders. You might be tempted to implement features like order cancellation, order editing, and order history, even though they're not part of the current requirements. Here's how applying the YAGNI principle can help you focus on what's important:
// Not adhering to YAGNI class OrderManager { createOrder() { // Implementation for creating an order } cancelOrder() { // Implementation for canceling an order - not needed yet } editOrder() { // Implementation for editing an order - not needed yet } getOrderHistory() { // Implementation for fetching order history - not needed yet } } // Adhering to YAGNI class OrderManager { createOrder() { // Implementation for creating an order } // Only add cancelOrder, editOrder, and getOrderHistory when they become necessary }
By following the YAGNI principle, you can focus on implementing the essential features, reducing complexity and development time. However, it's important to strike a balance between YAGNI and proper software design – you should still design your code with flexibility and maintainability in mind.
Composition Over Inheritance
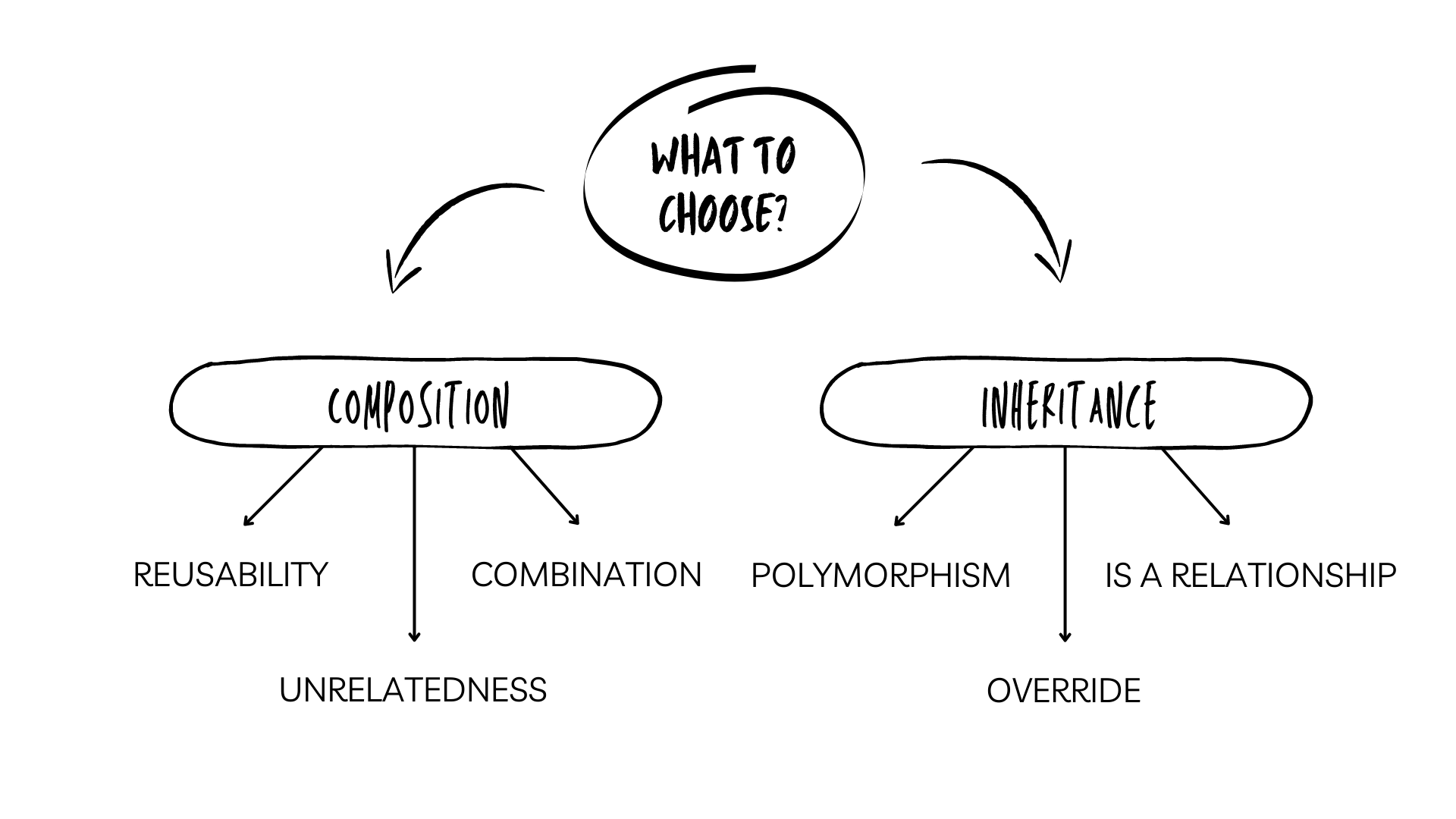
Definition: Composition Over Inheritance is a design principle that encourages the use of composition (combining simple objects to create more complex ones) instead of inheritance (creating new classes by inheriting properties and methods from a parent class) for code reuse and extensibility. This principle promotes flexibility and loose coupling in software design.
Benefits:
- Increased flexibility: Composition allows you to change or replace components at runtime, whereas inheritance is static and can't be changed after the class is defined.
- Reduced complexity: Composition reduces the depth of the inheritance hierarchy, making the codebase easier to understand and maintain.
- Better separation of concerns: Composition encourages creating smaller, more focused classes and components that are responsible for specific tasks.
When to use Composition:
- When you want to reuse functionality without being tied to a specific class hierarchy.
- When you need to combine different behaviors or functionalities that don't fit naturally in a single inheritance hierarchy.
- When the classes you want to combine have unrelated responsibilities or functionalities.
When to use Inheritance:
- When there's a clear "is-a" relationship between classes, and the derived class is a specific type of the base class.
- When you need to reuse or override specific functionality provided by the parent class.
- When you want to leverage polymorphism and dynamic dispatch to substitute objects based on their type.
Example:
Suppose you're building a game with various types of characters that have different abilities, such as walking, flying, or swimming. Here's how you can apply Composition Over Inheritance to create flexible and reusable components:
// Using Composition class Walks { walk() { console.log("I can walk"); } } class Flies { fly() { console.log("I can fly"); } } class Swims { swim() { console.log("I can swim"); } } class Character { constructor(abilities) { this.abilities = abilities; } performAbilities() { this.abilities.forEach((ability) => { ability(); }); } } const walkingCharacter = new Character([new Walks()]); walkingCharacter.performAbilities(); // Output: "I can walk" const flyingSwimmingCharacter = new Character([new Flies(), new Swims()]); flyingSwimmingCharacter.performAbilities(); // Output: "I can fly", "I can swim" // Using Inheritance class Character { walk() { console.log("I can walk"); } } class FlyingCharacter extends Character { fly() { console.log("I can fly"); } } class SwimmingCharacter extends Character { swim() { console.log("I can swim"); } }
By following the Composition Over Inheritance principle, you can create a more flexible, maintainable, and scalable codebase. It's important to evaluate each scenario carefully and choose the appropriate approach (composition or inheritance) based on the specific requirements and relationships between the classes.
Law of Demeter (Principle of Least Knowledge)
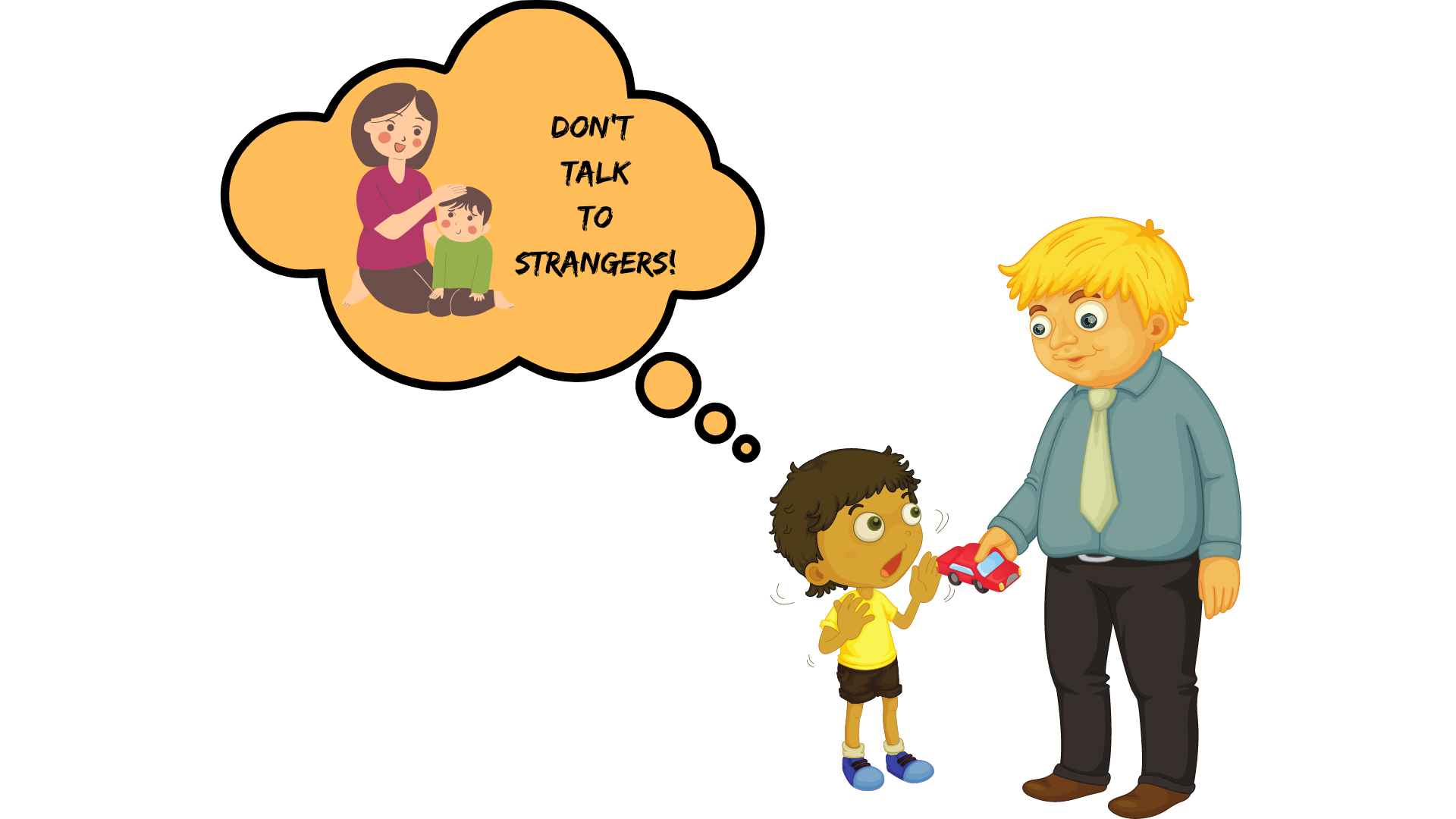
Definition: The Law of Demeter is a design principle that encourages reducing the coupling between components in a software system. It states that an object should only communicate with its immediate neighbors and should have limited knowledge about the structure and properties of other objects. By following the Law of Demeter, you can create a codebase that is easier to maintain and less prone to bugs.
Benefits:
- Easier maintenance: Loosely coupled code is easier to modify, as changes in one component have limited impact on others.
- Better encapsulation: By limiting the knowledge an object has about others, you promote better encapsulation and hide implementation details.
- Increased modularity: The Law of Demeter encourages dividing your code into smaller, more focused modules that have a single responsibility.
Guidelines to apply the Law of Demeter:
- Minimize the number of classes an object interacts with.
- Avoid "train-wreck" expressions, where you chain multiple method calls or property accesses together.
- Use dependency injection to provide an object with the resources it needs, rather than letting it create or locate those resources itself.
- Create well-defined interfaces between components to ensure that they only communicate through specific methods or properties.
- Favor method delegation over direct property access to reduce coupling between objects.
Example:
Suppose you're building a blogging platform, and you have classes representing users, posts, and comments. Here's how you can apply the Law of Demeter to create a better design:
// Not adhering to the Law of Demeter class User { getPosts() { // Implementation for fetching user posts } } class Post { getComments() { // Implementation for fetching post comments } } class Blog { getUser() { // Implementation for fetching a user } getRecentComments(userId) { const user = this.getUser(userId); const posts = user.getPosts(); const recentComments = posts.map((post) => post.getComments()).flat(); // Further processing to get recent comments } } // Adhering to the Law of Demeter class User { // ... } class Post { // ... } class CommentService { getRecentComments(user) { // Implementation for fetching recent comments for a user } } class Blog { constructor(commentService) { this.commentService = commentService; } getUser() { // Implementation for fetching a user } getRecentComments(userId) { const user = this.getUser(userId); const recentComments = this.commentService.getRecentComments(user); // Further processing to get recent comments } }
By following the Law of Demeter, you can create code that is easier to maintain, modify, and scale. Keep in mind that it's essential to strike a balance between following the Law of Demeter and over-engineering your solution. In some cases, adhering too strictly to the principle can result in overly complex and less readable code.
Conclusion
As you advance in your software development journey, it's essential to understand and appreciate the importance of software design principles like SOLID, YAGNI, KISS, DRY, and others. These principles act as a roadmap to creating well-structured, maintainable, and efficient software systems. By following these guidelines, you will be better equipped to write code that is easy to comprehend, modify, and extend, ultimately leading to enhanced software quality and adaptability.
We encourage you to explore more resources at DesignGurus.io to deepen your understanding of these software design principles and broaden your knowledge in system design.
Design Gurus offers comprehensive courses on software design to help you excel in your career. You can find these courses at the following links:
- Grokking the Object Oriented Design Interview
- Grokking the System Design Interview
- Grokking the Advanced System Design Interview
By leveraging these resources, you will not only create software that is more robust, maintainable, and efficient but also contribute to better products, improved user experiences, and long-term success in your professional career. These courses will equip you with essential skills and techniques to tackle complex system design challenges, making you a valuable asset in the software development field.
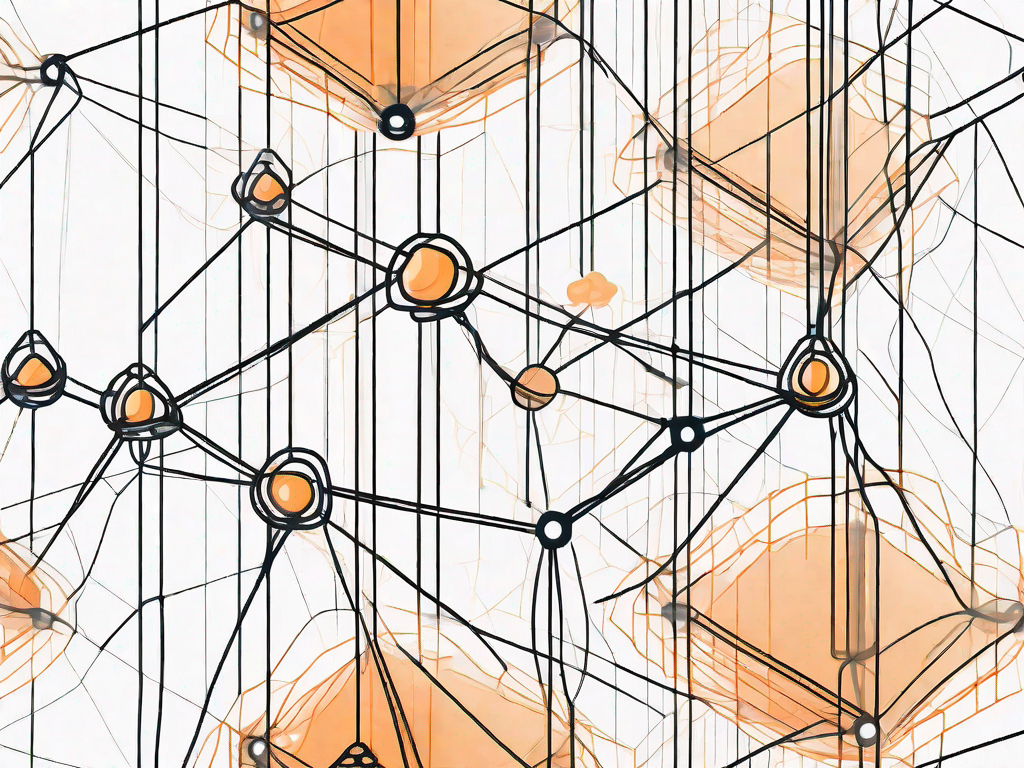
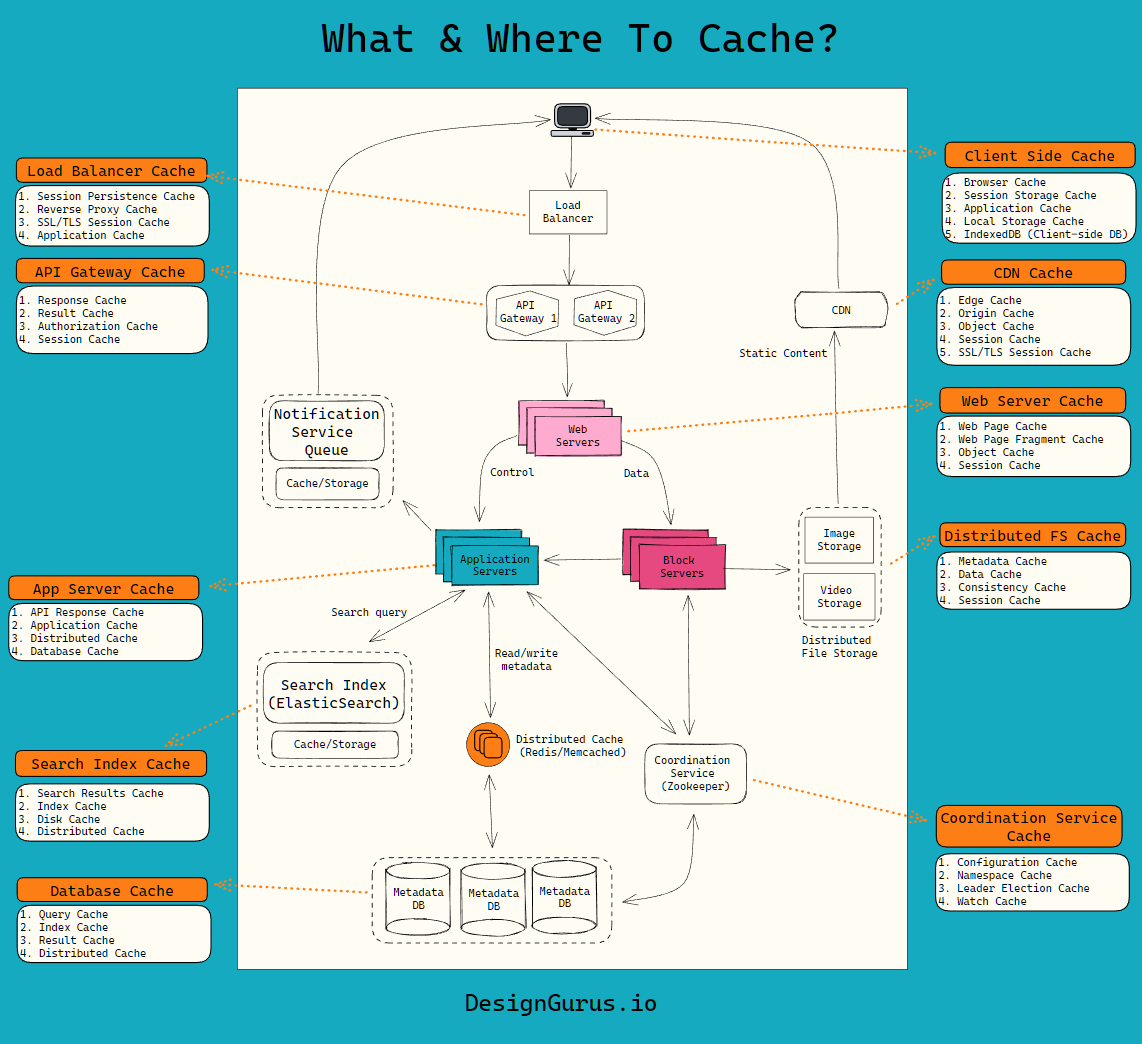
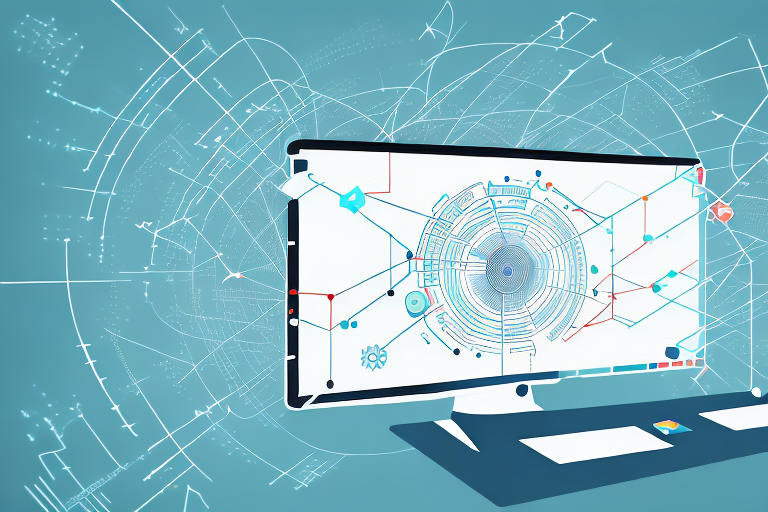
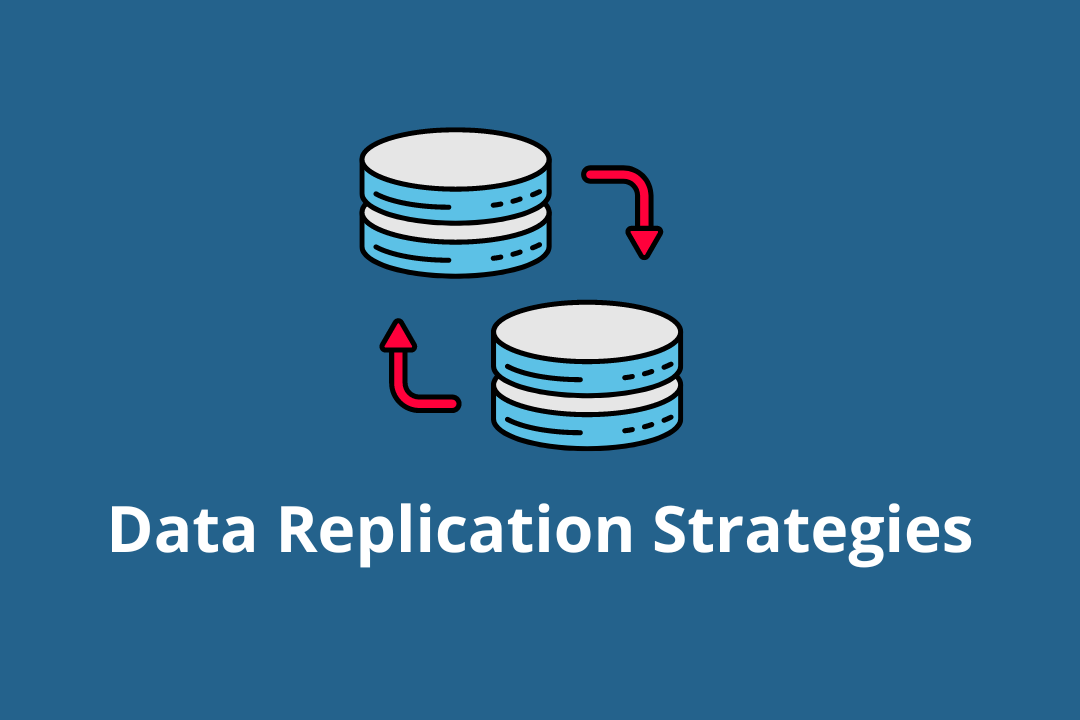
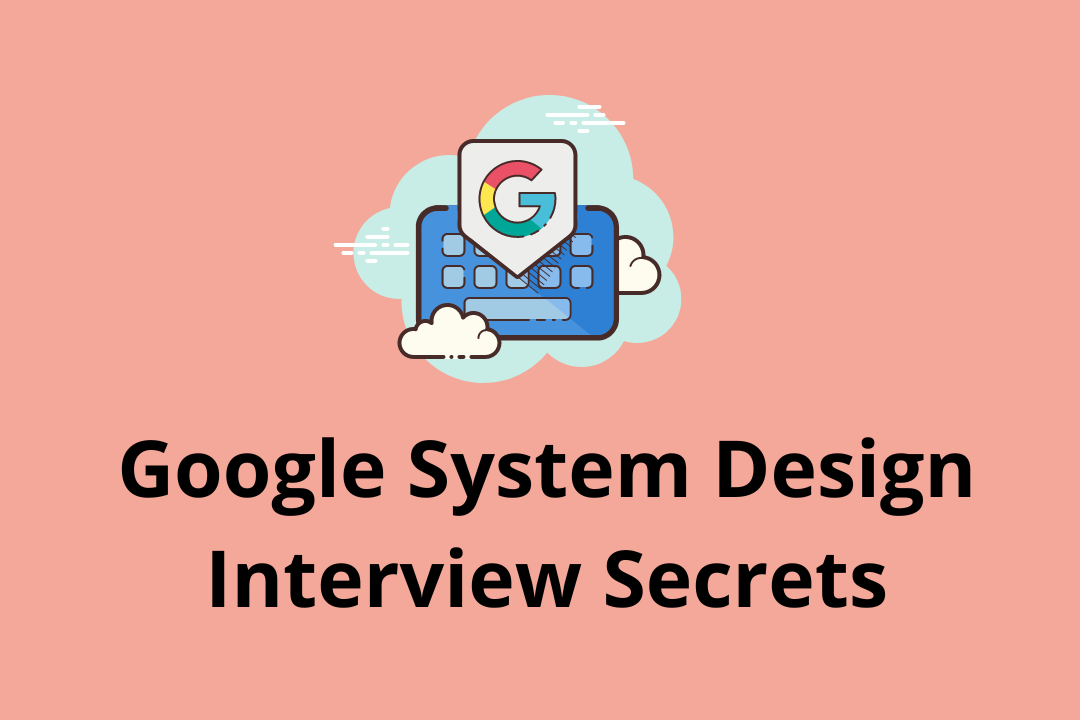
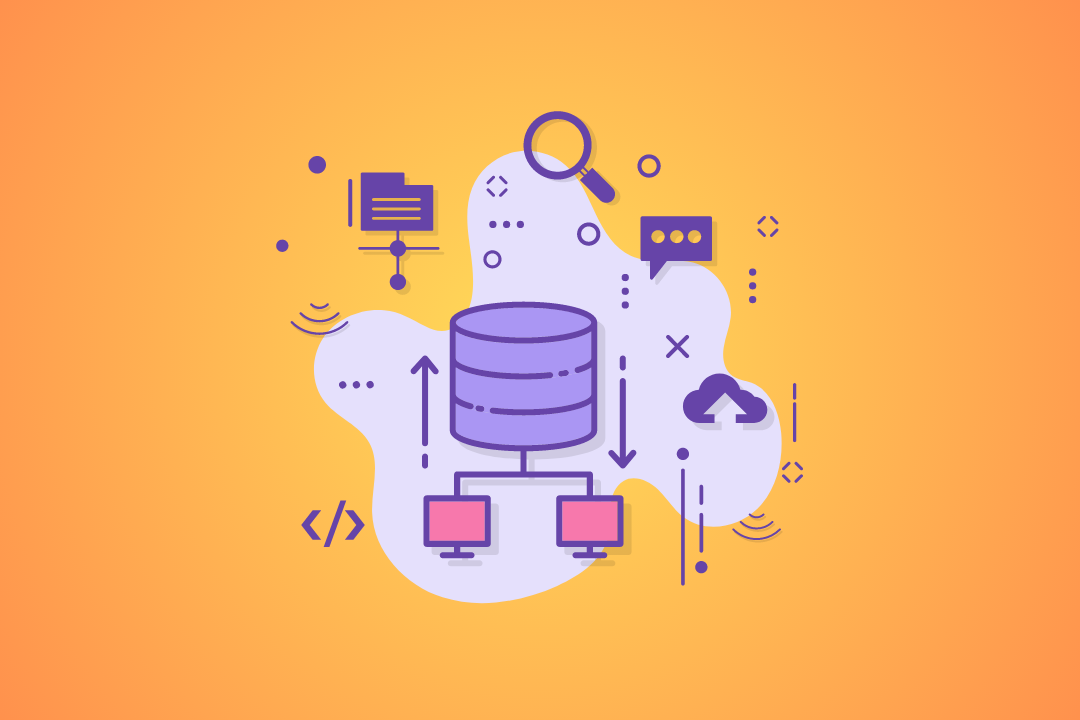