What is Java Map: A Complete Guide to Map Classes and Their Uses
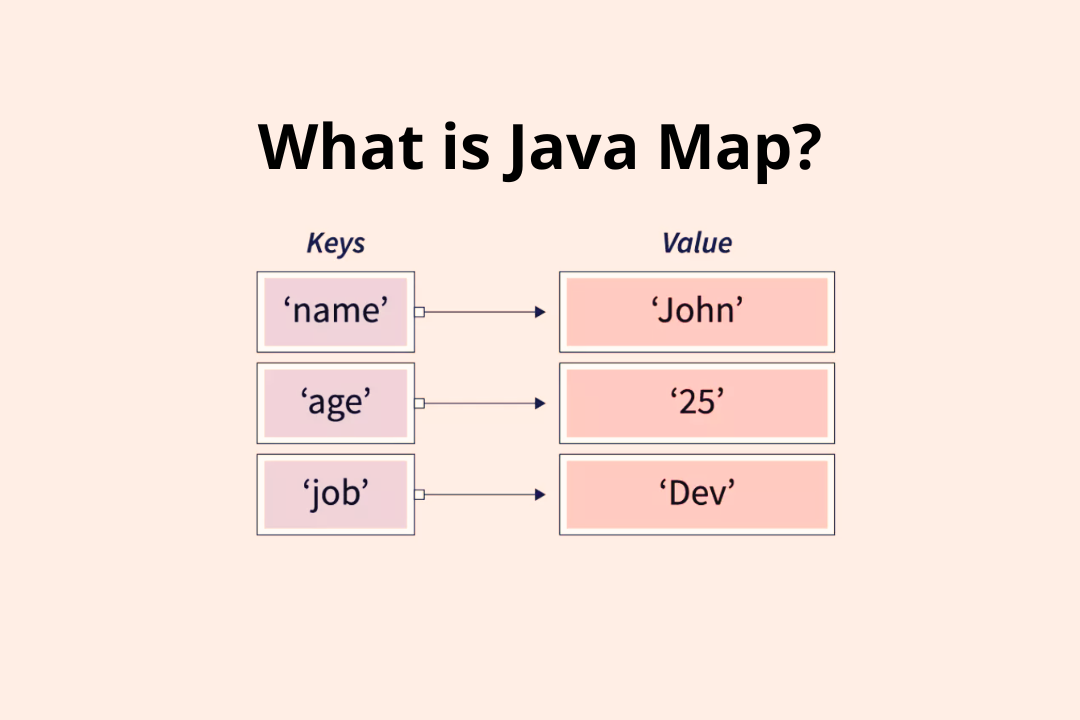
Java Map is a collection interface that provides a way to store data in a key-value format.
With Java Map, developers can quickly access data stored within the collection by its unique key, rather than iterating through a list to find the desired value. This makes it a popular and efficient way for developers to structure their data.
In this article, we'll dive into the basics of Java Map and explore the different types of Map classes available in the Java library. We'll also look at the benefits and advantages of using Java Map, and provide practical examples to help you utilize Java Map for maximum efficiency.
Understanding the Basics of Java Map
If you're new to Java programming, understanding the basics of Java Map can be a bit confusing. But don't worry, this article will provide a complete overview.
First, it's important to understand that Java Map is a data structure that allows you to store key-value pairs. Think of it as a dictionary where each word has a corresponding definition. In Java Map, the keys are unique identifiers and the values can be any Java object.
One of the most useful features of Java Map is that it allows you to quickly access a value based on its corresponding key. This can be incredibly helpful when working with large amounts of data.
It's worth noting that Java Map is an interface, which means that it can't be instantiated directly. Instead, you'll need to use one of the many Map classes available in the Java library.
One of the most commonly used Map classes is HashMap. This class provides a fast and efficient way to store and retrieve key-value pairs. It's also worth noting that HashMap does not guarantee the order of its elements.
Another commonly used Map class is TreeMap. This class is similar to HashMap, but it sorts its elements based on their keys. This can be useful in situations where you need to iterate over the elements in a specific order.
Finally, there's LinkedHashMap. This class is similar to HashMap, but it maintains the order in which its elements were inserted. This can be useful in situations where you need to preserve the order of your data.
Overall, Java Map is an incredibly powerful data structure that can be used in a wide variety of applications. Whether you're working with small amounts of data or large datasets, Java Map can help you store and retrieve your information quickly and efficiently.
Exploring the Different Types of Map Classes
As mentioned earlier, there are several Map classes available in the Java library. Each class has its unique features and benefits, making it essential to choose the right one for your specific needs. Here's a breakdown of some of the most commonly used Map classes:
HashMap
HashMap is the most commonly used Map class due to its fast performance and ease of use. It's an unordered collection that stores key-value pairs in a hash table.
One of the benefits of using HashMap is that it allows for quick retrieval of values based on their keys. This is because the hash function used to store the values in the table is designed to minimize collisions, which can slow down the retrieval process.
Another benefit of using HashMap is that it allows for null values and null keys. This means that developers can store and retrieve values without having to worry about whether or not they exist.
TreeMap
TreeMap is a sorted collection that stores key-value pairs in a red-black tree. It's useful when developers need to retrieve keys and values in a specific order, such as sorting by key value.
One of the benefits of using TreeMap is that it maintains the order of the keys, which can be useful in scenarios where developers need to retrieve values in a specific order. Additionally, TreeMap allows for null values, but not null keys. This means that developers can store and retrieve values, but they must ensure that the keys exist.
One potential drawback of using TreeMap is that it can be slower than other Map classes, such as HashMap, when retrieving values. This is because the red-black tree used to store the values is designed to maintain order, which can slow down the retrieval process.
LinkedHashMap
LinkedHashMap is similar to HashMap, but it maintains the insertion order of the keys. This makes it useful for scenarios where developers need to maintain the order of elements added to the Map.
One of the benefits of using LinkedHashMap is that it allows for quick retrieval of values based on their keys, similar to HashMap. However, it also maintains the order of the keys, which can be useful in scenarios where developers need to retrieve values in the order they were added.
Another benefit of using LinkedHashMap is that it allows for null values and null keys, similar to HashMap. This means that developers can store and retrieve values without having to worry about whether or not they exist.
Overall, the choice of Map class depends on the specific needs of the developer. If fast performance and ease of use are the top priorities, then HashMap is likely the best choice. If maintaining order is important, then LinkedHashMap or TreeMap may be the better choice, depending on whether or not null values or keys are allowed.
Unlocking the Benefits of Java Map
Java Map is a powerful tool that provides developers with a wide range of benefits. In this article, we will explore some of the key advantages of using Java Map and how it can help you organize and access data more efficiently.
Efficient Data Access
One of the most significant benefits of Java Map is its ability to provide efficient data access. This means that accessing data within a Map is much faster than iterating through a list to find a specific value. With Java Map, you can quickly retrieve the data you need, making it an ideal solution for applications that require real-time data retrieval.
For example, if you are building a stock market application that needs to retrieve real-time stock prices, Java Map can help you retrieve this data quickly and efficiently. This can help improve the overall performance of your application and provide a better user experience.
Flexible Key Value Pairing
Another significant advantage of Java Map is its flexible key value pairing. Java Map allows developers to associate any object with a unique key. This enables data to be structured and accessed in a way that suits the developer's unique needs.
For instance, if you are building a customer relationship management system, you can use Java Map to associate customer data with a unique identifier. This will enable you to quickly retrieve customer data based on their unique identifier, making it easier to manage and organize your customer data.
No Duplicate Keys
Java Map doesn't allow duplicate keys, ensuring that there's no ambiguity when data is being accessed or modified. This means that you can be confident that the data you are retrieving is accurate and up-to-date.
For example, if you are building a voting application, you can use Java Map to ensure that each vote is unique and that there are no duplicate votes. This can help ensure the integrity of your voting system and provide a fair and accurate representation of the votes.
Scalability
Java Map can handle large amounts of data and is optimized for quick data retrieval, making it a scalable and efficient solution for developers. This means that you can use Java Map to manage and organize large datasets without compromising on performance.
For instance, if you are building a social media application, you can use Java Map to manage and organize user data, such as user profiles, posts, and comments. This can help improve the overall performance of your application and provide a better user experience.
Conclusion
Java Map is a powerful tool that provides developers with a wide range of benefits. From efficient data access to flexible key value pairing, Java Map is an ideal solution for managing and organizing data. Whether you are building a small application or a large-scale system, Java Map can help you improve the performance and scalability of your application.
Exploring the Advantages of Using Map Classes
Maps are an essential data structure in programming, and they are used to store a collection of key-value pairs. Each Map class has its unique advantages and use cases, making it important to choose the right Map class for your needs. Here are some advantages of each of the Map classes we discussed:
HashMap
HashMap is one of the most commonly used Map classes in Java. It is a part of the Java Collections Framework and is implemented using a hash table. One of the primary advantages of HashMap is its fast performance due to its hash table implementation. HashMap is efficient in adding and removing elements, which makes it an ideal choice for large datasets. Additionally, HashMap allows null keys and values, which can be useful in some cases.
TreeMap
TreeMap is another Map class in Java that is implemented using a Red-Black tree. One of the primary advantages of TreeMap is that its elements are sorted by key value. This means that the TreeMap is always sorted, which makes it efficient for sorted operations such as min(), max(), and navigableMap(). TreeMap is also efficient for range queries and operations, which makes it an ideal choice for datasets that require such operations.
LinkedHashMap
LinkedHashMap is a Map class in Java that maintains the order of key-value pairs based on insertion order. One of the primary advantages of LinkedHashMap is that it is faster than TreeMap and maintains the order of elements. LinkedHashMap allows O(1) access to any element, which makes it an efficient choice for datasets that require frequent access to elements. LinkedHashMap is also useful when the order of elements is important.
Choosing the right Map class for your needs is essential in programming. By understanding the advantages and use cases of each Map class, you can make an informed decision on which Map class to use in your program.
Finding the Right Map Class for Your Needs
When it comes to storing and accessing data in Java, Map classes are an essential tool. They allow you to associate keys with values, creating a powerful data structure that can be used in a wide variety of applications. However, with so many different Map classes to choose from, it can be difficult to know which one is right for your needs.
One important factor to consider is the size of your data. If you have a large amount of data to store, you may want to choose a Map class that is optimized for performance with large datasets. HashMap, for example, is known for its fast access times and is a good choice for large, unordered datasets.
Another factor to consider is the typical usage scenario for your data. If you frequently need to retrieve data in a specific order, you may want to choose a Map class that can maintain that order. TreeMap, for example, is a sorted Map class that can be used to retrieve elements in a specific order.
But what if you need to maintain the order of elements while still maintaining fast access times? In that case, LinkedHashMap may be the right choice for you. This Map class maintains a linked list of the elements in the order they were inserted, while still providing fast access times for individual elements.
Ultimately, the choice of Map class will depend on your specific use case and the requirements of your project. Take some time to consider your needs and the characteristics of each Map class before making a decision.
How to Use Java Map for Maximum Efficiency
Java Map is a powerful tool for storing and manipulating data. It allows you to store key-value pairs and perform operations such as searching, inserting, and deleting elements. However, to ensure maximum efficiency when working with Java Map, there are several best practices to keep in mind:
- Choose the Right Map Class: As discussed earlier, it's important to choose the right Map class for your specific use case. For example, if you need to maintain the order of elements, you can use LinkedHashMap. On the other hand, if you don't need to maintain order but want faster access, you can use HashMap.
- Limit Resizing: Resizing a Map can be an expensive operation, so try to limit the number of elements added to the Map to avoid resizing it frequently. You can do this by setting an initial capacity when creating the Map.
- Use ConcurrentHashMap: ConcurrentHashMap is a thread-safe Map implementation that's useful in high-concurrency environments. It allows multiple threads to access and modify the Map concurrently without the risk of data corruption.
- Use Immutable Maps: Immutable Maps provide an efficient way to store data that doesn't need to be modified. They're useful for scenarios where data is mostly read, and write operations are infrequent. Immutable Maps can be created using the Guava library or Java 9's Map.of() method.
Another best practice when working with Java Map is to use the entrySet() method to iterate over the Map's key-value pairs. This method returns a Set of Map.Entry objects, which can be used to access both the key and the value of each element in the Map.
It's also important to keep in mind that Java Map is not suitable for all use cases. If you need to perform complex operations such as range searches or sorting, you may want to consider using a different data structure such as a tree-based Map or a database.
By following these best practices and choosing the right Map class for your use case, you can ensure maximum efficiency when working with Java Map.
Utilizing Java Map for Data Structuring
Java Map is an excellent way to structure data for fast and efficient access. Here are some ways Map classes can be used:
- Configurations: Store configuration settings, such as database connection details, in a Map for quick and easy access.
- Caching: Use a Map to store frequently accessed data for quick retrieval and to improve system performance.
- Lookup tables: Create a Map to store lookup tables for data that gets accessed in a predictable pattern.
Constructing a Java Map Step-by-Step
Creating a Java Map is a straightforward process that can be completed in just a few steps:
- Choose the appropriate Map implementation class.
- Create an instance of the Map using the appropriate constructor.
- Add key value pairs to the Map using the put() method.
- Access values using keys using the get() method.
- Remove key value pairs using the remove() method.
Troubleshooting Common Issues with Java Map
Working with Java Map can come with its share of issues. Here are some common issues and solutions:
- Duplicate Keys: Java Map doesn't allow duplicate keys. If you try to add a key that already exists, the value will overwrite the existing value.
- Null Keys and Values: Some Map implementations allow null keys and values, while others do not. Be sure to check the documentation for the specific Map implementation you're using.
- Resizing: Resizing a Map can be an expensive operation, so try to limit the number of elements added to the Map to avoid resizing it frequently.
Practical Examples of Java Map Usage
Finally, let's look at some practical examples of how Java Map can be used:
- Storing User Information: Use a Map to store user information such as name, email address, and password.
- Storing Product Information: Store product information such as price, description, and availability in a Map for easy access and sorting.
- Storing Stock Information: Track stock levels using a Map to quickly see which products need restocking.
Conclusion
Java Map is a powerful tool for structuring and accessing data in a fast and efficient manner. In this article, we've explored the basics of Java Map, the different types of Map classes available in the Java library, and the benefits and advantages of using Java Map. We've also provided best practices and practical examples to help you utilize Java Map for maximum efficiency. By following these guidelines and taking advantage of Java Map's functionality, you can streamline your application's data structure and make it more efficient and effective in meeting your needs.
➡ To learn about system design basics, have t a look at Grokking System Design Fundamentals.
➡ Learn more on architecture and system design in Grokking the System Design Interview and Grokking the Advanced System Design Interview.
Read more on system design interview.
[1] 18 System Design Concepts Every Engineer Must Know Before the Interview.
[2] Top LeetCode Patterns for FAANG Coding Interviews
[3] The Complete Guide to Ace the System Design Interview
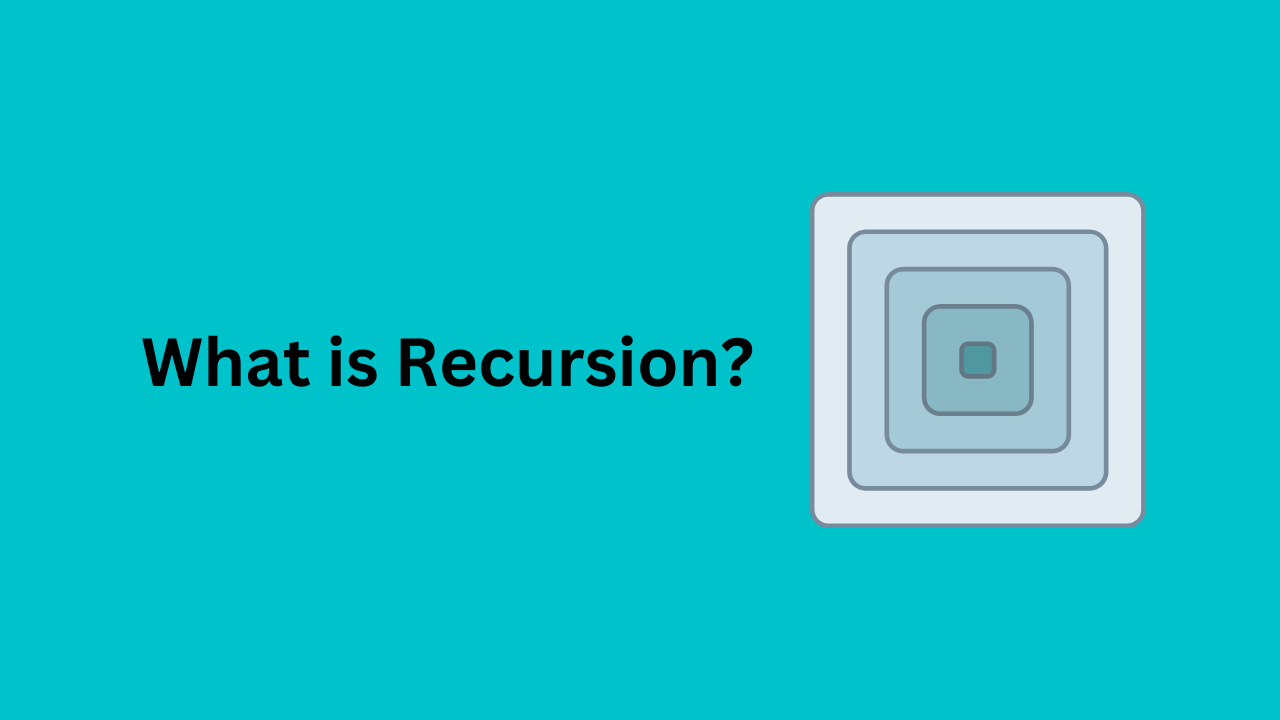
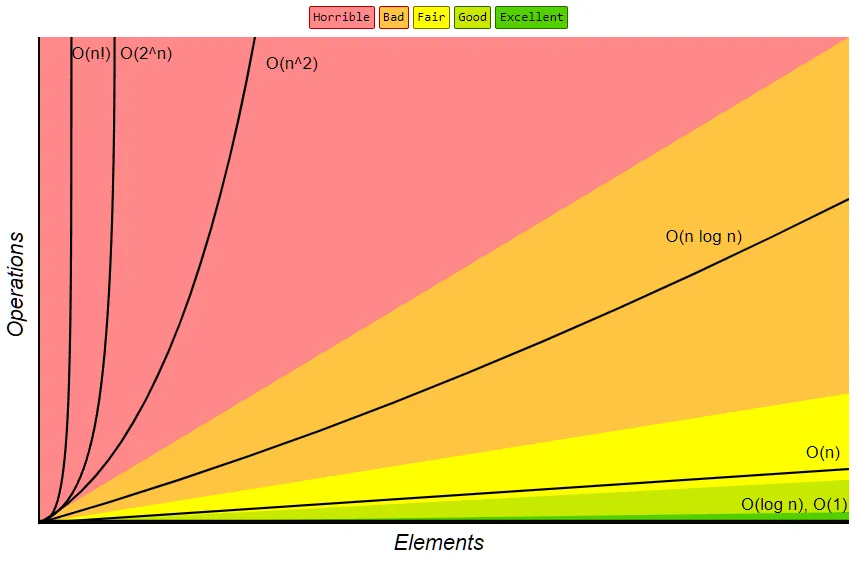
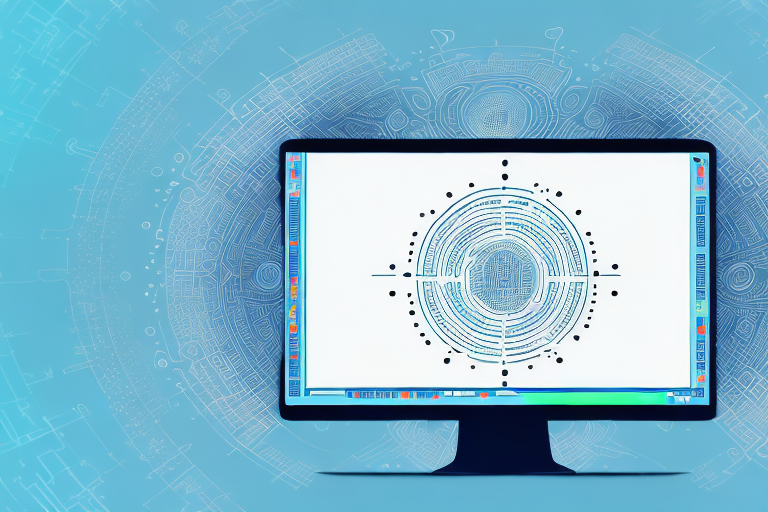