0% completed
Problem Statement
Write a function to calculate the nth Fibonacci number.
Fibonacci numbers are a series of numbers in which each number is the sum of the two preceding numbers. First few Fibonacci numbers are: 0, 1, 1, 2, 3, 5, 8, ...
Mathematically we can define the Fibonacci numbers as:
Fib(n) = Fib(n-1) + Fib(n-2), for n > 1 Given that: Fib(0) = 0, and Fib(1) = 1
Constraints:
0 <= n <= 30
Basic Solution
A basic solution could be to have a recursive implementation of the mathematical formula discussed above:
The time complexity of the above algorithm is exponential O(2^n) as we are making two recursive calls in the same function. The space complexity is O(n) which is used to store the recursion stack.
Let's visually draw the recursion for CalculateFibonacci(4)
to see the overlapping subproblems:
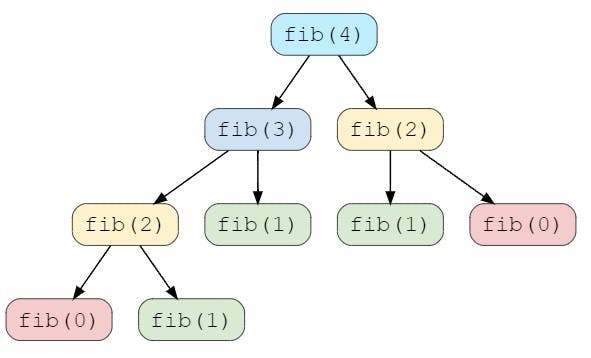
We can clearly see the overlapping subproblem pattern: fib(2)
has been called twice and fib(1)
has been called thrice. We can optimize this using memoization to store the results for subproblems.
Top-down Dynamic Programming with Memoization
We can use an array to store the already solved subproblems. Here is the code:
Bottom-up Dynamic Programming
Let's try to populate our dp[]
array from the above solution, working in a bottom-up fashion. Since every Fibonacci number is the sum of the previous two numbers, we can use this fact to populate our array.
Here is the code for the bottom-up dynamic programming approach:
The above solution has time and space complexity of O(n).
Memory optimization
We can optimize the space used in our previous solution. We don't need to store all the Fibonacci numbers up to 'n', as we only need two previous numbers to calculate the next Fibonacci number. We can use this fact to further improve our solution:
The above solution has a time complexity of O(n) but a constant space complexity O(1).