0% completed
Problem Statement
Given two strings 's1' and 's2', find the length of the longest substring which is common in both the strings.
Example 1:
Input: s1 = "abdca"
s2 = "cbda"
Output: 2
Explanation: The longest common substring is "bd".
Example 2:
Input: s1 = "passport"
s2 = "ppsspt"
Output: 3
Explanation: The longest common substring is "ssp".
Constraints:
- 1 <= s1.length, s2.length <= 1000`
s1
ands2
consist of only lowercase English characters.
Basic Solution
A basic brute-force solution could be to try all substrings of 's1' and 's2' to find the longest common one. We can start matching both the strings one character at a time, so we have two options at any step:
- If the strings have a matching character, we can recursively match for the remaining lengths and keep a track of the current matching length.
- If the strings don't match, we start two new recursive calls by skipping one character separately from each string and reset the matching length.
The length of the Longest Common Substring (LCS) will be the maximum number returned by the three recurse calls in the above two options.
Code
Here is the code:
Because of the three recursive calls, the time complexity of the above algorithm is exponential O(3^{m+n}), where 'm' and 'n' are the lengths of the two input strings. The space complexity is O(m+n), this space will be used to store the recursion stack.
Top-down Dynamic Programming with Memoization
We can use an array to store the already solved subproblems.
The three changing values to our recursive function are the two indexes (i1 and i2) and the 'count'. Therefore, we can store the results of all subproblems in a three-dimensional array. (Another alternative could be to use a hash-table whose key would be a string (i1 + "|" i2 + "|" + count)).
Code
Here is the code:
Bottom-up Dynamic Programming
Since we want to match all the substrings of the given two strings, we can use a two-dimensional array to store our results. The lengths of the two strings will define the size of the two dimensions of the array. So for every index 'i' in string 's1' and 'j' in string 's2', we have two options:
- If the character at
s1[i]
matchess2[j]
, the length of the common substring would be one plus the length of the common substring tilli-1
andj-1
indexes in the two strings. - If the character at the
s1[i]
does not matchs2[j]
, we don't have any common substring.
So our recursive formula would be:
if s1[i] == s2[j] dp[i][j] = 1 + dp[i-1][j-1] else dp[i][j] = 0
Let's draw this visually for "abcda" and "cbda". Starting with a substring of zero lengths, if any one of the string has zero length, then the common substring will be of zero length:
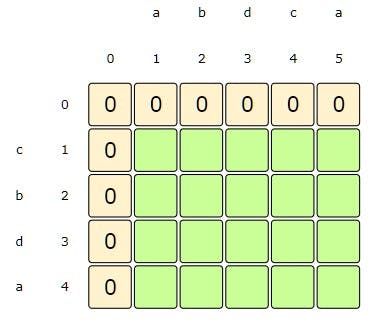
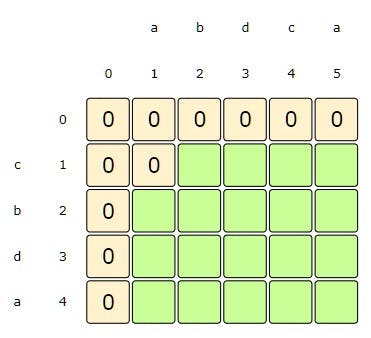
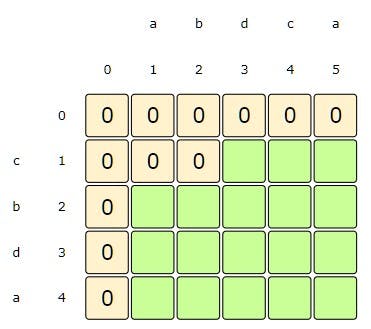
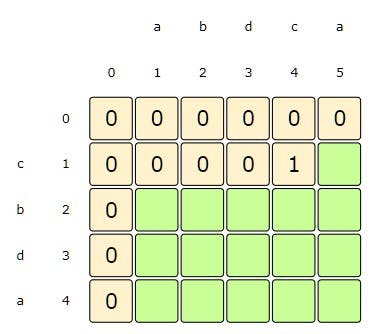
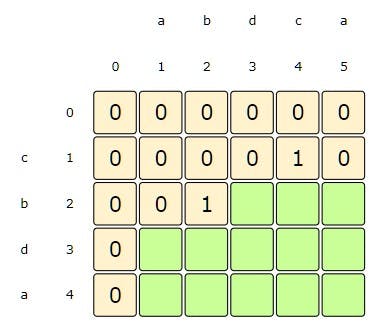
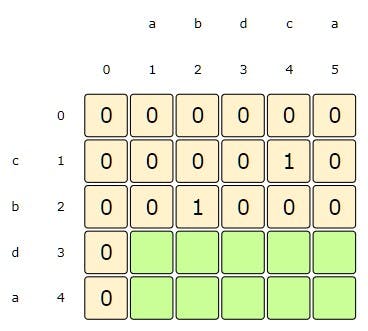
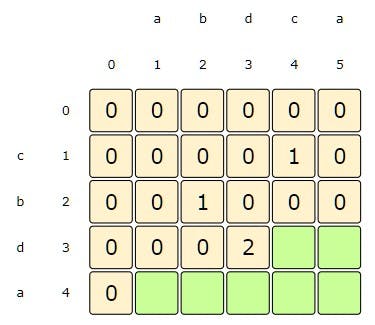
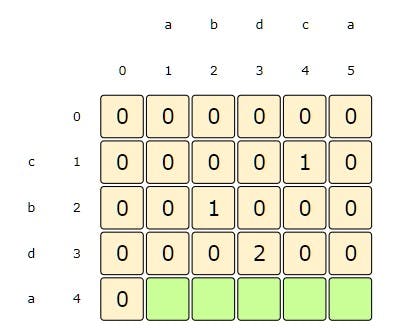
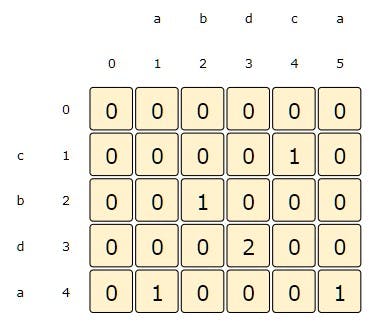
From the above visualization, we can clearly see that the longest common substring is of length '2'-- as shown by dp[3][3]
.
Here is the code for our bottom-up dynamic programming approach:
The time and space complexity of the above algorithm is O(m*n), where 'm' and 'n' are the lengths of the two input strings.
Challenge
Can we further improve our bottom-up DP solution? Can you find an algorithm that has O(n) space complexity?
Hint
We only need one previous row to find the optimal solution!