7 Coding Interview Challenges and How to Crack Them
Coding is a challenging task—and preparing for coding interviews can be more daunting.
People often don't know what to prepare and expect from such interviews. But if you follow the right approach and understand the common challenges, you can significantly increase your chances of success.
In this blog, we'll look at some typical coding problems often asked in tech job interviews and offer practical advice on how to solve them.
This guide will help individuals land a job in the tech industry by brushing up on their coding skills. So, let's get started!
1. Array and String Manipulation
Array and string questions are a staple in coding interviews. They might ask you to reverse an array without using extra space or find the longest substring without repeating characters in a string.
Key Concepts
-
Indexing: Understand how to access and modify elements in arrays and strings via their indices.
-
Two-pointer techniques: Common in problems involving sorting, searching, or modifying arrays in-place.
-
String functions: Familiarize yourself with built-in string operations like substring, length, and conversion methods.
How To Prepare
-
Practice is key.
-
Get comfortable with manipulating indices and using temporary variables to swap values.
-
For strings, familiarize yourself with ASCII values and how you might use them to track occurrences of characters.
2. Binary Trees Problems
Whether it's finding the depth of a binary tree or performing a level-order traversal, questions about binary trees are common in interviews because they test your knowledge of data structures.
Key Concepts
-
Tree Traversals: Know how to perform in-order, pre-order, post-order, and level-order traversals.
-
Recursive Solutions: Most tree problems can be solved effectively using recursion.
-
Tree Properties: Understand concepts like tree height, balanced trees, and binary search trees.
How To Prepare
-
Understand the properties of trees and typical tree operations.
-
Practice recursive and iterative solutions for traversals.
-
Always check for edge cases like single-node trees or completely unbalanced trees.
Learn the 20 important coding patterns for your tech interview.
3. Dynamic Programming
Dynamic programming problems can range from calculating the nth Fibonacci number to finding the minimum path sum in a grid.
These problems are challenging because they require you to come up with a solution that is both correct and efficient in terms of time and space complexity.
Key Concepts
-
Memoization: Using extra space to store intermediate results of recursive calls.
-
Tabulation: An iterative approach with a table usually based on the size of the input.
-
Optimization Problems: Practice common patterns like finding the longest/shortest path, subset problems, and maximizing or minimizing certain conditions.
How To Prepare
-
Start by understanding the recursive solution and then think about how you can avoid recomputation using memoization or tabulation.
-
Solve as many different problems as you can to recognize patterns in problem-solving with dynamic programming.
4. Graph-Based Questions
Graphs are versatile data structures, and their questions might involve finding the shortest path, detecting cycles, or determining if a graph is bipartite.
Key Concepts
-
Graph Traversal Algorithms: Depth-first search (DFS) and breadth-first search (BFS) should be second nature.
-
Shortest Path Algorithms: Dijkstra’s and Bellman-Ford for weighted graphs, and BFS for unweighted graphs.
-
Cycle Detection: Know how to detect cycles in directed and undirected graphs using DFS or BFS.
How To Prepare
-
Learn about depth-first search (DFS), breadth-first search (BFS), and other graph algorithms.
-
Make sure you know how to implement them from scratch and can apply them to both directed and undirected graphs.
5. Sorting and Searching Algorithms
From implementing quicksort to binary search on a rotated array, sorting and searching are integral to solving more complex problems efficiently.
Key Concepts
-
Sorting Mechanisms: Understand quicksort, mergesort, heapsort, and when to use each.
-
Binary Search: Must be able to implement and understand its application beyond simple array searches (e.g., search in a rotated array or finding an entry in a matrix).
-
Complexity Analysis: Know the time and space complexities of common sorting and searching algorithms.
How To Prepare
-
Be thorough with each sorting algorithm’s mechanism, its efficiency, and its drawbacks.
-
For searching, understand when and how to apply binary search, even in less obvious situations.
Find more tips to navigate your coding interview.
6. Bit Manipulation
Bit manipulation problems might ask you to count the number of 1 bits in an integer or find a single number in an array where every element appears twice except for one.
Key Concepts
-
Bit Operations: AND, OR, XOR, NOT, and bit shifts (left and right).
-
Bit Tricks: Common techniques like using XOR to find an element appearing once in a list where others appear twice.
-
Use Cases: Practice using bit manipulation for compact storage and performance optimization.
How To Prepare
-
Learn the common bit operations like AND, OR, XOR, NOT, and bit shifting.
-
Practice using these to solve classic problems, as these can often lead to more optimal solutions in terms of space and time.
7. Concurrency Questions
Concurrency issues are crucial for jobs at companies where software performance and efficiency are critical.
You might be asked to implement a multithreaded producer-consumer setup or solve problems using semaphores.
Key Concepts
-
Basic Threading: Understand how to create and manage threads in the programming language of your choice.
-
Synchronization Tools: Know how to use mutexes, semaphores, and other synchronization primitives to handle common concurrency problems.
-
Concurrency Issues: Be aware of potential problems such as deadlocks, and race conditions, and how to avoid them.
How To Prepare
-
Understand basic concepts of threading and process synchronization.
-
Learn about the challenges of concurrent programming like deadlock, livelock, and race conditions.
For a detailed guide, check out the roadmap to clearing technical interviews in 2024.
Final Words
Coding interviews don't have to be scary. With targeted practice and a clear understanding of fundamental concepts, you can tackle any problem they throw at you.
Remember, consistency is key.
Regular practice can help you sharpen your skills and boost your confidence. Good luck with your coding interview preparation, and remember to keep learning and improving every day!
If you are struggling with finding the right resources to prepare for tech interviews like most people, check out the power-packed coding interview courses by DesignGurus.io.
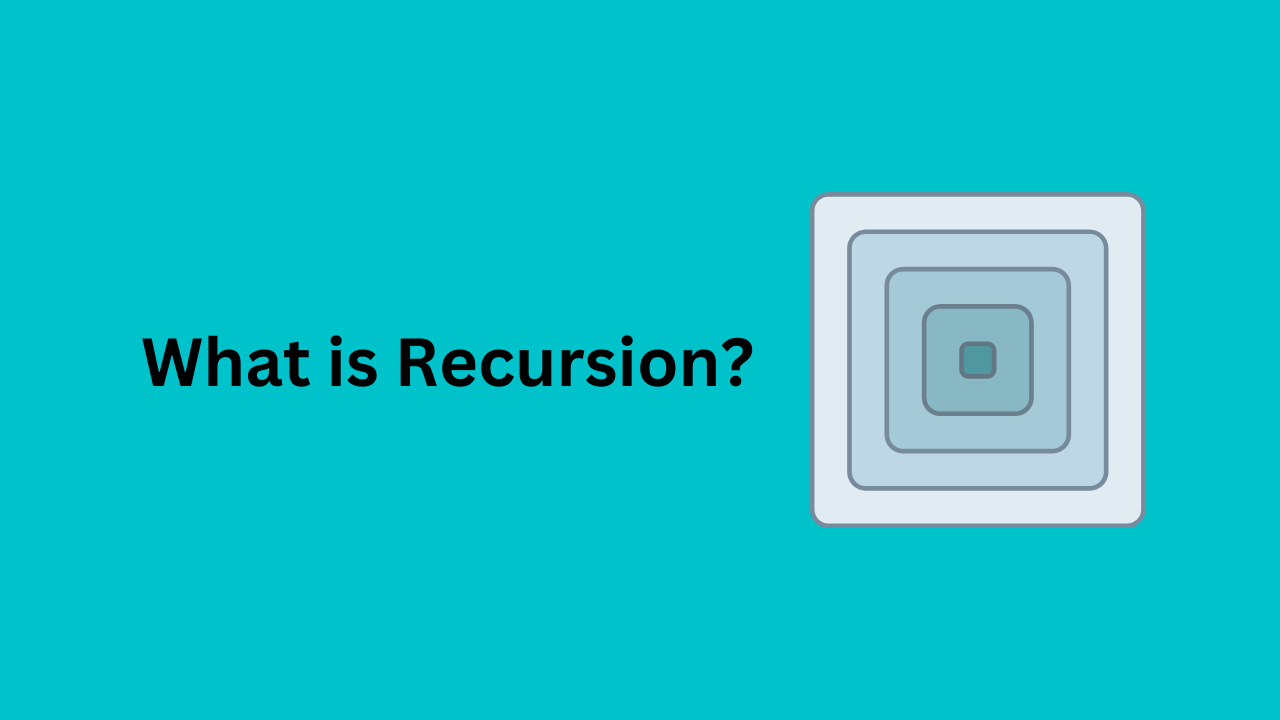
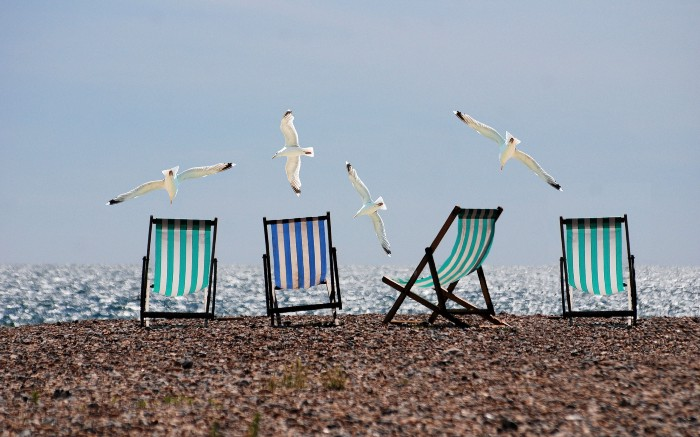
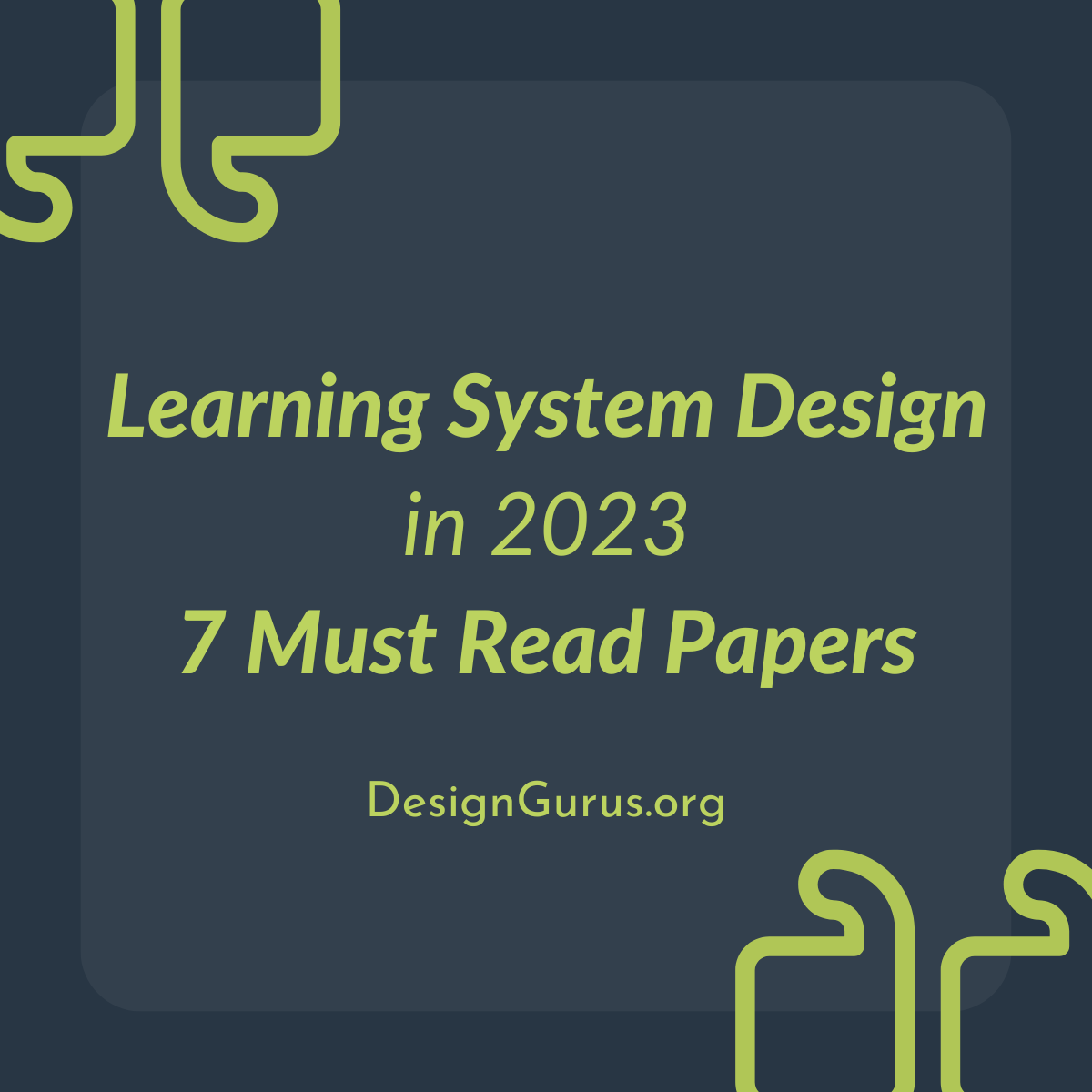
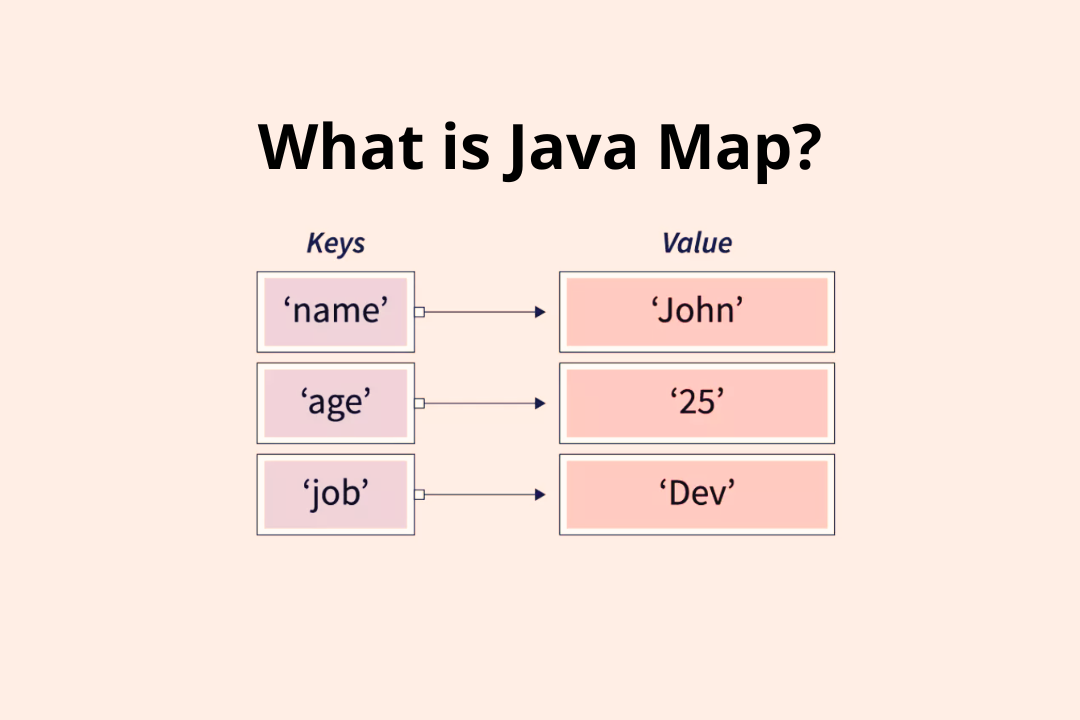