Top 3 ChatGPT Prompts To Learn Any LeetCode Coding Pattern
Leetcode is the biggest online repository of coding problems for programmers to polish their skills.
Learning to tackle coding problems on Leetcode is a key skill for anyone preparing for software engineering and technical interviews.
With ChatGPT, you can master any Leetcode coding patterns simply by giving effective prompts that will help you learn and practice the most common coding patterns used on Leetcode.
Let us cover the top three ChatGPT prompts you can use to learn any Leetcode coding patterns. We will be discussing the sliding-window Leetcode coding pattern in this guide.
ChatGPT Prompt 1
Explain the sliding window coding pattern in simple words for beginners. Tell me where is this coding pattern used and what type of problems does it solve? Also, discuss its characteristics and how can we identify this pattern.
ChatGPT Response
The "Sliding Window" pattern is a versatile technique used in algorithmic problems, especially those involving sequences like arrays or strings. It's particularly efficient for problems that require examining a subset of elements at a time, such as a continuous sequence or a subarray of a specific length.
Where is the Sliding Window Used
The sliding window technique is primarily used with problems that deal with arrays or strings where you need to:
- Find maximum/minimum sums or lengths of subarrays/substrings under certain constraints.
- Track frequencies of elements within a certain range in the array or substring.
Types of Problems Solved by Sliding Window
- Maximum/minimum subarray problems: These might involve finding the maximum sum of all subarrays of a given size, or the longest substring without repeating characters.
- Substring problems: Such as finding the shortest substring that contains all the characters of a set.
- Contiguous array problems: For example, finding the longest contiguous subarray with elements that fulfill a specific property (like a sum that equals a given number).
Characteristics of the Sliding Window Pattern
- Variable or Fixed Size: The window might have a fixed size (sliding the window involves moving both the start and end forward by one element) or a variable size (the end expands to include more elements and the start moves forward to shrink the window).
- Two Pointers: This pattern often utilizes two pointers (representing the beginning and end of the window) that can move independently.
- Efficiency: It provides a way to reduce the time complexity from O(n^2) (which would be the case with nested loops) to O(n) by eliminating the need for redundant processing.
How to Identify the Sliding Window Pattern
You can recognize when a problem might benefit from the sliding window approach by the following indicators:
- The problem involves a subarray or substring: If it's about finding a subarray or substring that meets certain criteria, a sliding window is often suitable.
- Optimization is related to a range: Problems asking for optimization over a continuous range, like maximum sum or longest/shortest length under certain constraints, are key candidates.
- Input size vs. output requirement: If the problem mentions a large input size but requires efficient computation (hinting at linear time complexity), the sliding window might be the way to go.
- Incremental calculation: If each element of the array or string only needs to be processed once as the window expands or contracts, it's a sign that the sliding window could be efficient.
ChatGPT Prompt 2
Please give me 5 problems that can be solved using this sliding window coding pattern. Divide the problems into easy, intermediate, and hard levels. Also, explain why should these coding problems be solved?
ChatGPT Response
The Sliding Window technique is quite effective for a range of problems that deal with contiguous sequences or substrings within arrays or strings.
Here’s a breakdown of five such problems across different difficulty levels that are typically found on platforms like LeetCode, explaining why these problems are beneficial to solve:
Easy Problems
1. Maximum Sum Subarray of Size K (LeetCode 643 - Maximum Average Subarray I)
Problem Statement: Given an array consisting of n integers, find the contiguous subarray of given length k that has the maximum average value.
Why To Solve?
This problem helps us understand how to maintain a sum over a sliding window and how to adjust the window by incorporating one new element while removing the oldest one. This foundation is crucial for grasping more complex sliding window problems.
2. Minimum Size Subarray Sum (LeetCode 209)
Problem Statement:
Given an array of positive integers and a positive integer s, find the minimal length of a contiguous subarray of which the sum ≥ s.
Why To Solve?
It's an excellent problem to practice the variable size sliding window technique, where the window size isn't fixed, and you learn to expand and contract the window based on runtime conditions.
Intermediate-Level Problems
3. Longest Substring Without Repeating Characters (LeetCode 3)
Problem Statement: Given a string, find the length of the longest substring without repeating characters.
Why To Solve?
This problem teaches how to manage and update a window while tracking elements inside it using a HashSet or HashMap. It's fundamental for understanding how to handle substrings and unique characters efficiently.
4. Sliding Window Maximum (LeetCode 239)
Problem Statement: You are given an array of integers nums, there is a sliding window of size k which is moving from the very left of the array to the very right. You can only see the k numbers in the window. Each time the sliding window moves right by one position. Return the max sliding window.
Why To Solve?
This problem is crucial for understanding complex window operations and is often solved with a deque (double-ended queue) to efficiently track the maximum in the current window, showcasing a more advanced use of the sliding window with auxiliary data structures.
Hard Problems
5. Minimum Window Substring (LeetCode 76)
Problem Statement: Given two strings s and t, return the minimum window in s which will contain all the characters in t.
Why To Solve?
This problem is a classic example that utilizes a sliding window to find the smallest substring covering all desired characters. It is particularly useful for learning about character frequency counting, expanding and contracting the window based on those counts, and balancing multiple conditions to maintain the window's validity.
This is a higher-level application of sliding windows that incorporates multiple aspects of hashing, string manipulation, and window adjustments.
ChatGPT Prompt 3
Help me write Python executable code for two problems mentioned above using sliding window approach with comments. Also, explain its working.
ChatGPT Response
Sure! Below is a Python code implementing the sliding window technique with comments explaining its working. We'll solve the problem of finding the maximum sum of a subarray of size 'k' within an array of integers.
How it Works
-
We initialize max_sum to negative infinity to ensure that it gets updated with the maximum sum found during traversal.
-
We initialize window_sum to 0, which will represent the sum of the elements within the sliding window.
-
We iterate through the array 'nums'.
-
For each element, we add it to window_sum.
-
Once the window size reaches 'k', we check if the current window_sum is greater than max_sum.
-
If it is, we update max_sum with the value of window_sum.
-
Then, we remove the leftmost element from the window by subtracting nums[i - k + 1] from window_sum.
-
Finally, we return max_sum, which contains the maximum sum of a subarray of size 'k' within the array.
This code efficiently finds the maximum sum of a subarray of size k using the sliding window technique. It maintains a window of size k as it traverses the array, updating the window sum and the maximum sum as necessary.
This approach has a time complexity of O(n), making it efficient for large arrays.
Example Code 2:
How it Works
-
We initialize char_set to an empty set to store unique characters encountered in the substring.
-
We initialize max_length to 0, which will hold the length of the longest substring without repeating characters.
-
We initialize two pointers, 'left' and 'right', to represent the left and right ends of the sliding window.
-
We iterate through the string s using the right pointer.
-
If the character at the right pointer is not in the char_set, we add it to the set, update max_length if needed, and expand the window to the right (right += 1).
-
If the character is already in the char_set, indicating a repeating character, we remove the character at the left pointer from the set and slide the window to the right (left += 1).
-
We continue this process until the 'right' pointer reaches the end of the string.
-
Finally, we return max_length, which contains the length of the longest substring without repeating characters.
This code efficiently finds the length of the longest substring without repeating characters using the sliding window technique. It maintains a window of unique characters as it traverses the string, updating the maximum length as necessary.
This approach has a time complexity of O(n), making it efficient for large strings.
Wrapping Up
LeetCode provides hundreds of problems covering many topics that are crucial for coding interviews. It helps users learn different approaches and techniques.
This post covers prompts ranging from common patterns in coding interviews and can help build your problem-solving skills effectively.
Practice them regularly, and you'll be better prepared for your next coding interview!
Read interesting Leetcode guide or find top Leetcode patterns.
To ace your technical interview in the first attempt, join special courses for interview preparation with DesignGurus.io
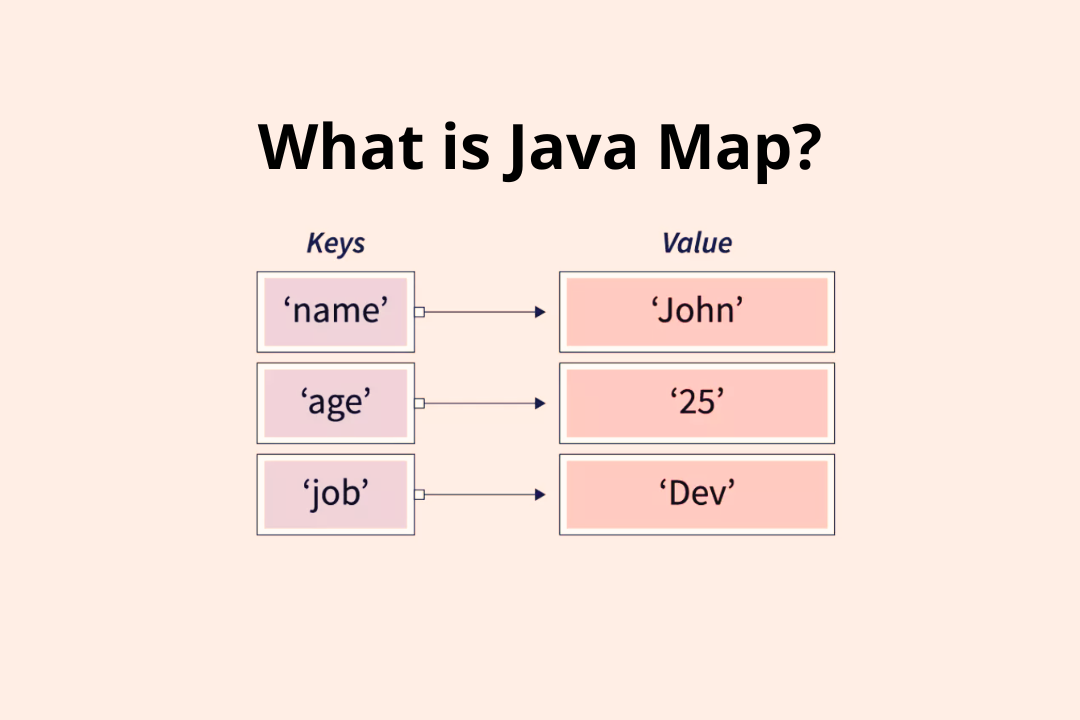
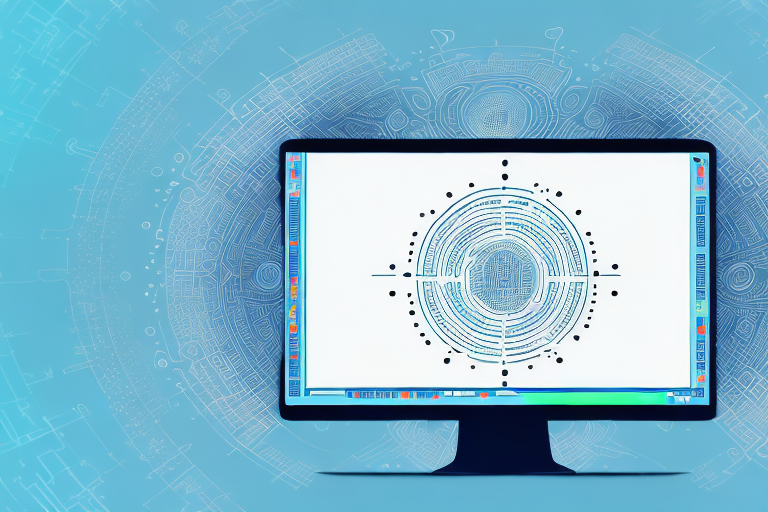
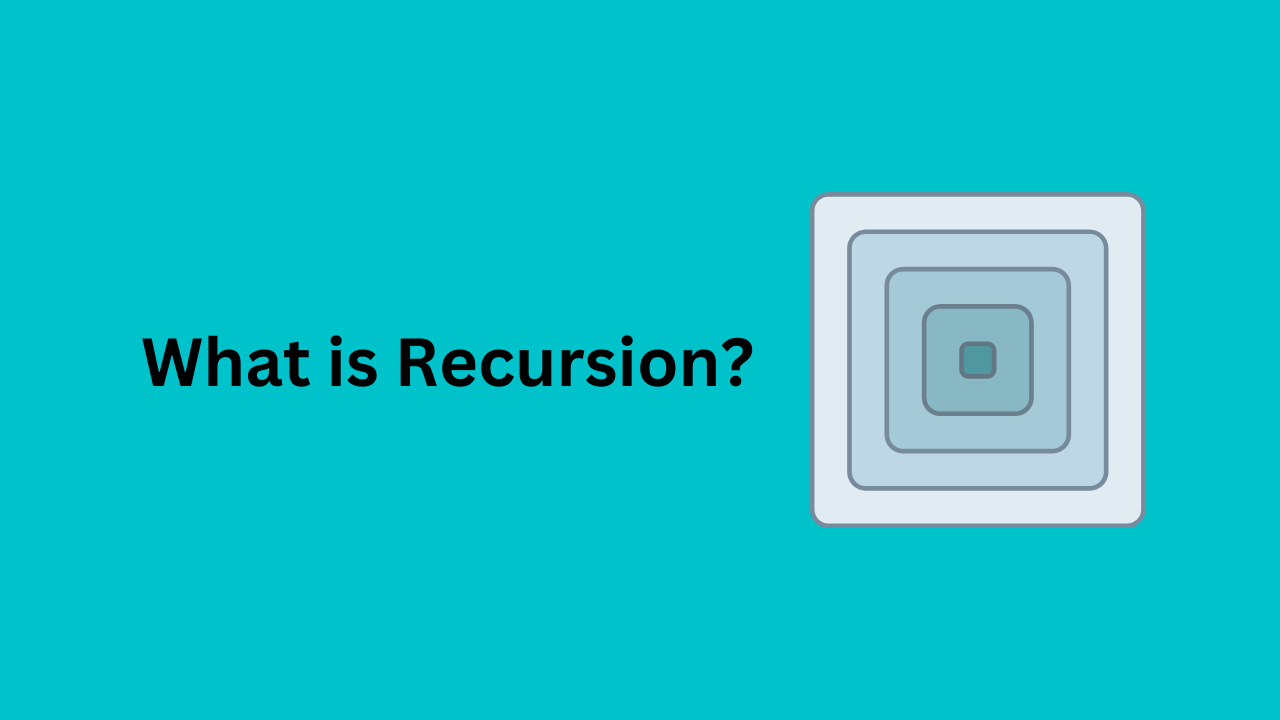